编写一段程序,使用管道来实现父子进程之间的进程通信c++
时间: 2024-10-12 22:04:27 浏览: 33
在C++中,可以使用`pipe()`系统调用来创建管道,然后通过fork()函数创建父子进程,利用管道来进行进程间通信。这里是一个简单的例子,展示如何在C++中实现基本的管道通信:
```cpp
#include <iostream>
#include <unistd.h>
#include <sys/types.h>
// 父进程
void parent(int fd[2]) {
char message[] = "Hello from parent!";
// 关闭不需要的管道描述符
close(fd[0]);
// 向管道写入数据
write(fd[1], message, strlen(message) + 1);
std::cout << "Parent process wrote to pipe." << std::endl;
}
// 子进程
void child(int fd[2]) {
char buffer[100];
// 关闭不需要的管道描述符
close(fd[1]);
// 从管道读取数据
read(fd[0], buffer, sizeof(buffer));
buffer[sizeof(buffer) - 1] = '\0'; // 结束字符串
std::cout << "Child process received: " << buffer << std::endl;
// 等待一段时间让父进程看到结果
sleep(1);
std::cout << "Child process exiting..." << std::endl;
}
int main() {
int pipe_fds[2];
if (pipe(pipe_fds) == -1) {
perror("Failed to create pipe");
return 1;
}
pid_t pid = fork();
if (pid == 0) { // 子进程
child(pipe_fds);
exit(0);
} else if (pid > 0) { // 父进程
parent(pipe_fds);
wait(NULL); // 等待子进程结束
} else {
perror("Fork failed");
return 1;
}
return 0;
}
```
在这个例子中,父进程向管道写入一条消息,子进程从管道读取并打印出来。注意,为了保证数据完整性,我们通常会关闭不需要的管道描述符,并在读取和写入结束后手动关闭它们。
阅读全文
相关推荐
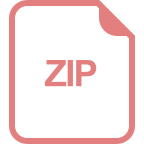
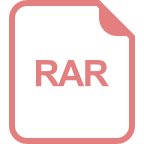
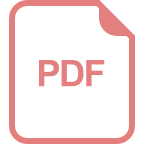
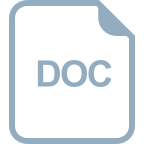
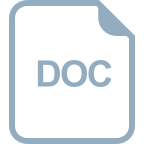
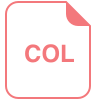
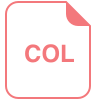
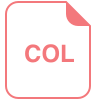
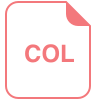
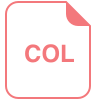
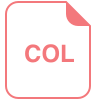
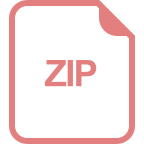
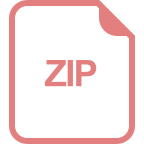
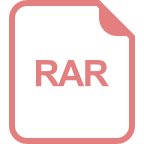
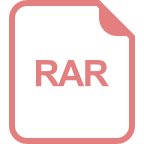
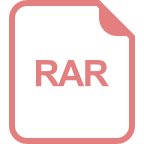
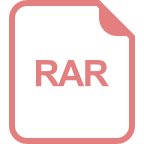
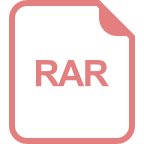