用Java通过输入输出流实现仿记事本程序,能够实现新建、打开、保存、另存等基本功能,给我整个程序的完整代码
时间: 2024-12-11 15:28:05 浏览: 12
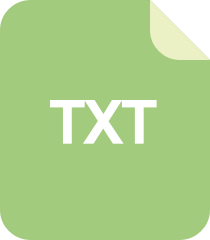
能够掌握常用组件的基本操作以及输入输出流的使用
在Java中,通过`java.io`包中的`InputStreamReader`、`OutputStreamWriter`以及`FileInputStream`、`FileOutputStream`等类可以实现简单的文本文件操作,模拟记事本程序的基本功能。以下是实现这个功能的一个简化版示例:
```java
import java.io.*;
public class SimpleNoteEditor {
private File currentFile;
public void start() {
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 新建");
System.out.println("2. 打开");
System.out.println("3. 保存");
System.out.println("4. 另存为");
System.out.println("5. 退出");
try {
int choice = Integer.parseInt(Console.readLine());
switch (choice) {
case 1:
createNewFile();
break;
case 2:
openExistingFile();
break;
case 3:
saveCurrentFile();
break;
case 4:
saveAsNewFile();
break;
case 5:
exitProgram();
return;
default:
System.out.println("无效选择,试试看其他选项吧!");
}
} catch (NumberFormatException e) {
System.out.println("请输入数字选择!");
}
}
}
// 新建文件
private void createNewFile() {
if (currentFile != null) {
System.out.println("已存在当前文件,请先关闭再新建!");
return;
}
String filename = askForFilename();
currentFile = new File(filename);
Files.write(currentFile.toPath(), "".getBytes());
}
// 打开文件
private void openExistingFile() {
String filename = askForFilename();
try {
currentFile = new File(filename);
if (!currentFile.exists()) {
System.out.println("文件不存在!");
return;
}
Files.readAllBytes(currentFile.toPath()).forEach(System.out::print);
} catch (IOException e) {
System.out.println("读取文件失败:" + e.getMessage());
}
}
// 保存文件
private void saveCurrentFile() {
if (currentFile == null) {
System.out.println("尚未创建或打开文件,无法保存!");
return;
}
try {
Files.write(currentFile.toPath(), Files.readAllBytes(currentFile.toPath()));
} catch (IOException e) {
System.out.println("保存文件失败:" + e.getMessage());
}
}
// 另存为
private void saveAsNewFile() {
String newFilename = askForFilename();
try {
File newFile = new File(newFilename);
Files.write(newFile.toPath(), Files.readAllBytes(currentFile.toPath()));
currentFile = newFile;
} catch (IOException e) {
System.out.println("另存为失败:" + e.getMessage());
}
}
// 询问用户输入文件名
private String askForFilename() {
System.out.print("请输入文件名:");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
return br.readLine();
}
// 退出程序
private void exitProgram() {
currentFile = null; // 清理资源
System.exit(0);
}
public static void main(String[] args) {
SimpleNoteEditor editor = new SimpleNoteEditor();
editor.start();
}
}
阅读全文
相关推荐
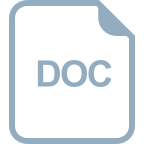
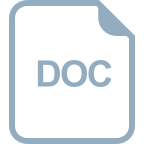

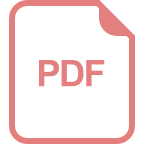
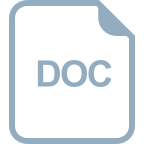
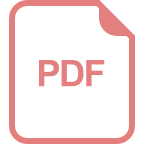
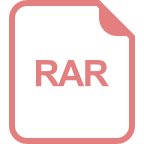
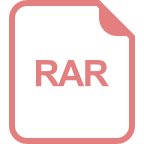
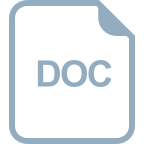
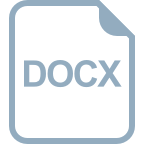
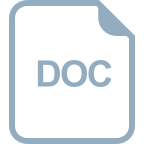
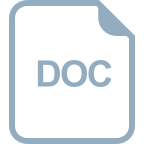
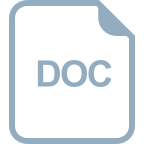
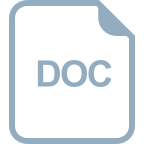
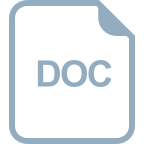
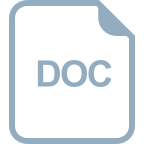
