treewidget 鼠标右击选项时显示菜单栏
时间: 2024-09-14 17:06:09 浏览: 15
在Qt框架中,如果你想要在使用`QTreeWidget`时在鼠标右击事件触发时显示菜单栏(通常称为上下文菜单),你可以通过重写`QTreeWidget`的`contextMenuEvent`方法来实现。以下是一个简单的例子:
```cpp
#include <QTreeWidget>
#include <QMenu>
#include <QAction>
#include <QContextMenuEvent>
class MyTreeWidget : public QTreeWidget {
public:
MyTreeWidget(QWidget *parent = nullptr) : QTreeWidget(parent) {
// 初始化菜单项
QAction *action1 = new QAction("Action 1", this);
QAction *action2 = new QAction("Action 2", this);
// 将菜单项添加到一个菜单中
QMenu *menu = new QMenu(this);
menu->addAction(action1);
menu->addAction(action2);
// 设置上下文菜单
this->setContextMenuPolicy(Qt::CustomContextMenu);
// 连接信号和槽
connect(this, SIGNAL(customContextMenuRequested(const QPoint&)), this, SLOT(showContextMenu(const QPoint&)));
}
public slots:
void showContextMenu(const QPoint& point) {
QTreeWidgetItem *item = this->itemAt(point);
// 这里可以根据item来定制菜单项
if (item) {
QMenu *menu = new QMenu(this);
QAction *action1 = new QAction("Delete", this);
connect(action1, &QAction::triggered, this, [this, item](){
// 这里添加删除item的代码
this->takeTopLevelItem(this->indexOfTopLevelItem(item));
});
menu->addAction(action1);
menu->exec(this->mapToGlobal(point));
}
}
};
```
在上面的代码中,我们首先创建了一个自定义的`QTreeWidget`类,然后重写了`showContextMenu`槽函数,该函数会在鼠标右击时被调用。我们使用`QMenu`来创建一个菜单,并添加了两个`QAction`对象。然后我们通过`setContextMenuPolicy(Qt::CustomContextMenu)`设置了上下文菜单策略,并将鼠标右击事件通过信号`customContextMenuRequested`与槽函数`showContextMenu`连接起来。
在`showContextMenu`函数中,我们首先获取了鼠标点击位置的`QTreeWidgetItem`对象,然后根据该对象来定制上下文菜单的内容。在这个例子中,我们只添加了一个"Delete"的菜单项,并为它绑定了一个槽函数,该槽函数会删除选中的树节点。
请确保在你的主窗口类中创建`MyTreeWidget`的实例,以使这段代码能够正常工作。
相关推荐
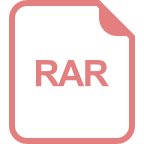
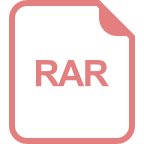
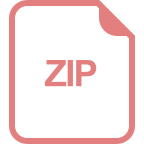





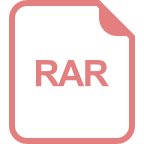
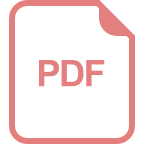
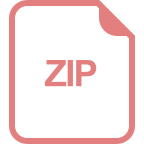
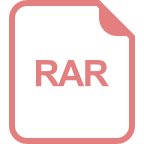
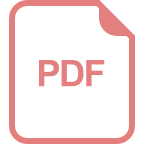
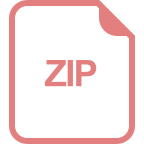
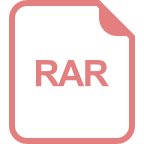
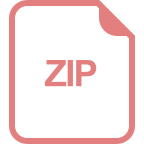