c++ qt widget 例程
时间: 2023-09-03 21:02:12 浏览: 135
Qt是一个跨平台的应用程序开发框架,提供了丰富的库和工具,用于开发图形用户界面和其他应用程序。Qt Widget是Qt框架中的一个模块,用于创建和管理图形界面元素,如窗口、按钮、输入框等。
在Qt中,使用Widget编写一个简单的例程非常简单。首先,我们需要创建一个Qt Widget项目。然后,在主窗口中添加各种需要的控件,例如按钮、文本框等。接下来,可以通过设置各个控件的属性和信号槽来实现交互逻辑。最后,编译并运行程序,即可看到创建的窗口和控件。
例如,我们可以编写一个计算器的例程。首先,在主窗口中放置一些按钮和一个文本框,用于输入和显示计算结果。然后,编写相应的函数来实现按钮的点击事件。例如,当用户点击数字按钮时,将该数字添加到文本框中;当用户点击运算符按钮时,将运算符添加到文本框中。最后,编写一个计算函数来实现实际的计算逻辑,将计算结果显示到文本框中。
通过以上步骤,我们就可以完成一个简单的Qt Widget例程。当用户运行程序时,会出现一个计算器界面,可以进行简单的数学运算。这只是一个简单的示例,Qt Widget可以用于开发各种类型的应用程序,例如文本编辑器、图像处理工具等。
总之,Qt Widget是Qt框架中用于创建图形用户界面的模块,通过添加控件、处理事件和信号槽来实现交互逻辑,可以用于开发各种功能丰富的应用程序。
相关问题
ubuntu系统下,使用qt creater,使图片输出成灰度图、降低分辨率的图片的main.cpp和mainwindow.cpp的例程,以及对应的.pro文件
以下是一个简单的例程,可以将图像转换为灰度图,并降低分辨率。需要在.pro文件中添加QT += widgets和QT += gui。
main.cpp
```c++
#include <QApplication>
#include "mainwindow.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
mainwindow.h
```c++
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QLabel>
#include <QImage>
#include <QSpinBox>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QPushButton>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void openImage();
void convertToGray();
void reduceResolution();
private:
QLabel *imageLabel;
QImage image;
QSpinBox *spinBox;
};
#endif // MAINWINDOW_H
```
mainwindow.cpp
```c++
#include "mainwindow.h"
#include <QFileDialog>
#include <QMessageBox>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
imageLabel = new QLabel;
imageLabel->setBackgroundRole(QPalette::Base);
imageLabel->setSizePolicy(QSizePolicy::Ignored, QSizePolicy::Ignored);
imageLabel->setScaledContents(true);
QWidget *centralWidget = new QWidget(this);
setCentralWidget(centralWidget);
QVBoxLayout *mainLayout = new QVBoxLayout(centralWidget);
QHBoxLayout *buttonLayout = new QHBoxLayout;
QPushButton *openButton = new QPushButton(tr("Open"), this);
QPushButton *grayButton = new QPushButton(tr("Convert to Gray"), this);
QPushButton *reduceButton = new QPushButton(tr("Reduce Resolution"), this);
spinBox = new QSpinBox(this);
spinBox->setRange(1, 10);
spinBox->setValue(1);
buttonLayout->addWidget(openButton);
buttonLayout->addWidget(grayButton);
buttonLayout->addWidget(reduceButton);
buttonLayout->addWidget(spinBox);
mainLayout->addWidget(imageLabel);
mainLayout->addLayout(buttonLayout);
connect(openButton, SIGNAL(clicked()), this, SLOT(openImage()));
connect(grayButton, SIGNAL(clicked()), this, SLOT(convertToGray()));
connect(reduceButton, SIGNAL(clicked()), this, SLOT(reduceResolution()));
}
MainWindow::~MainWindow()
{
}
void MainWindow::openImage()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("Open Image"), ".", tr("Image Files (*.png *.jpg *.bmp)"));
if (fileName.isEmpty())
return;
image.load(fileName);
if (image.isNull())
{
QMessageBox::information(this, tr("Error"), tr("Cannot load %1.").arg(fileName));
return;
}
imageLabel->setPixmap(QPixmap::fromImage(image));
}
void MainWindow::convertToGray()
{
if (image.isNull())
return;
image = image.convertToFormat(QImage::Format_Grayscale8);
imageLabel->setPixmap(QPixmap::fromImage(image));
}
void MainWindow::reduceResolution()
{
if (image.isNull())
return;
int factor = spinBox->value();
image = image.scaled(image.width() / factor, image.height() / factor);
imageLabel->setPixmap(QPixmap::fromImage(image));
}
```
.pro文件
```c++
QT += widgets gui
TARGET = imageConverter
TEMPLATE = app
SOURCES += main.cpp \
mainwindow.cpp
HEADERS += mainwindow.h
```
这个例程中,使用了Qt的几个基本控件,如QLabel、QSpinBox、QPushButton等,并且利用QImage类进行图像处理。openImage()函数用于打开图片,convertToGray()函数将图片转换为灰度图,reduceResolution()函数将图片降低分辨率。
阅读全文
相关推荐
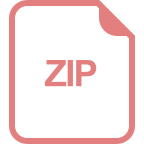
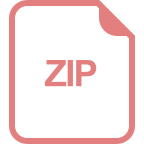
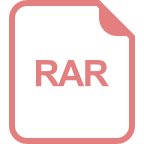
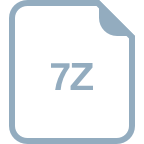
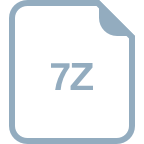
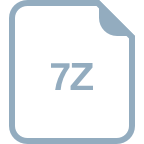
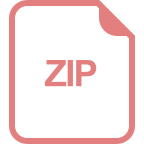
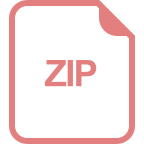
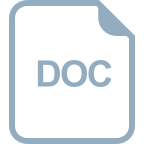
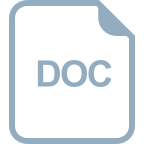
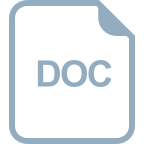
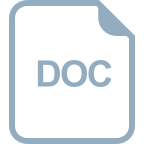
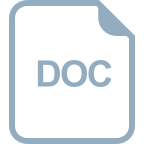
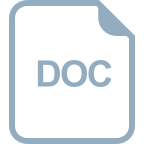
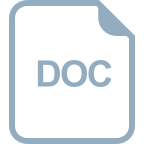