vue 3 ts [ECharts] There is a chart instance already initialized on the dom.
时间: 2023-10-19 18:29:41 浏览: 59
The error message "There is a chart instance already initialized on the DOM" in Vue 3 with TypeScript usually occurs when you try to initialize an ECharts chart on a DOM element that already has a chart instance attached to it.
To fix this issue, you need to ensure that you are not initializing multiple instances of ECharts on the same DOM element. Here are a few possible solutions:
1. Use the `dispose` method: Before reinitializing the chart, make sure to dispose of the existing instance using the `dispose` method. This will remove the previous chart and its event listeners from the DOM.
```typescript
import { ref, onBeforeUnmount } from "vue";
import * as echarts from "echarts";
export default {
setup() {
const chartRef = ref<HTMLDivElement | null>(null);
let chartInstance: echarts.ECharts | null = null;
const initData = () => {
if (chartRef.value && chartInstance) {
// Dispose of the existing chart instance
chartInstance.dispose();
}
// Initialize the new chart instance
chartInstance = echarts.init(chartRef.value);
// ...
};
onBeforeUnmount(() => {
if (chartInstance) {
chartInstance.dispose();
chartInstance = null;
}
});
return {
chartRef,
initData,
};
},
};
```
2. Use a flag to track initialization: Instead of creating a new chart instance every time, you can use a flag to track whether the chart has already been initialized. Only initialize the chart if it hasn't been initialized yet.
```typescript
import { ref, onMounted } from "vue";
import * as echarts from "echarts";
export default {
setup() {
const chartRef = ref<HTMLDivElement | null>(null);
let isChartInitialized = false;
const initData = () => {
if (!isChartInitialized && chartRef.value) {
// Initialize the chart instance
const chartInstance = echarts.init(chartRef.value);
isChartInitialized = true;
// ...
}
};
onMounted(initData);
return {
chartRef,
initData,
};
},
};
```
Ensure that you are calling the `initData` method at the appropriate time, such as when the component is mounted or when the data that the chart depends on changes.
I hope this helps! Let me know if you have any further questions.
相关推荐
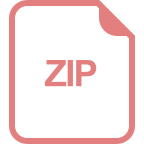














