java使用websocket聊天
时间: 2023-12-08 17:05:39 浏览: 30
以下是使用Java实现WebSocket聊天的步骤:
1. 首先需要导入Java WebSocket API,可以在Maven中添加以下依赖项:
```xml
<dependency>
<groupId>javax.websocket</groupId>
<artifactId>javax.websocket-api</artifactId>
<version>1.1</version>
</dependency>
```
2. 创建一个WebSocket服务器端点,继承`javax.websocket.Endpoint`类,并实现`onOpen`、`onClose`、`onError`和`onMessage`方法。在`onOpen`方法中,可以将新连接的会话添加到会话列表中。
```java
@ServerEndpoint("/chat")
public class ChatEndpoint {
private static final List<Session> sessions = new ArrayList<>();
@OnOpen
public void onOpen(Session session) {
sessions.add(session);
}
@OnClose
public void onClose(Session session) {
sessions.remove(session);
}
@OnError
public void onError(Session session, Throwable throwable) {
throwable.printStackTrace();
}
@OnMessage
public void onMessage(String message, Session session) throws IOException {
for (Session s : sessions) {
if (s.isOpen()) {
s.getBasicRemote().sendText(message);
}
}
}
}
```
3. 在`onMessage`方法中,将接收到的消息广播给所有连接的会话。
4. 创建一个WebSocket客户端,使用`javax.websocket.WebSocketContainer`接口的`connectToServer`方法连接到服务器端点。
```java
public class ChatClient {
public static void main(String[] args) throws Exception {
WebSocketContainer container = ContainerProvider.getWebSocketContainer();
Session session = container.connectToServer(new Endpoint() {
@Override
public void onOpen(Session session, EndpointConfig config) {
session.addMessageHandler(new MessageHandler.Whole<String>() {
@Override
public void onMessage(String message) {
System.out.println(message);
}
});
}
}, URI.create("ws://localhost:8080/chat"));
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
while (true) {
String message = reader.readLine();
session.getBasicRemote().sendText(message);
}
}
}
```
5. 在客户端中,使用`javax.websocket.Session`接口的`getBasicRemote`方法发送消息。
相关推荐
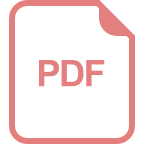














