创建一个单链表的插入和删除
时间: 2024-09-25 15:12:24 浏览: 30
创建单链表的插入和删除操作通常涉及节点的动态管理。这里我会简要讲解如何在头插法和尾插法以及删除特定节点的情况下实现这些操作。
**1. 插入操作:**
- **头插法(Insert at beginning):**
- 首先,创建新节点,并将其 `next` 指针设置为当前链表的头节点;
- 然后更新头节点指针,使其指向新节点。
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
def insert_at_beginning(head, data):
new_node = Node(data)
new_node.next = head
head = new_node
return head
```
- **尾插法(Insert at end):**
- 如果链表为空,直接将新节点设为头结点;
- 否则,遍历到链表尾部,即最后一个节点,将其 `next` 指向新节点。
```python
def insert_at_end(head, data):
if not head:
return Node(data)
current = head
while current.next:
current = current.next
current.next = Node(data)
return head
```
**2. 删除操作:**
- **删除指定值(Delete a node with a specific value):**
- 遍历链表找到目标节点;
- 如果找到了,更新前一个节点的 `next` 指针跳过目标节点。
```python
def delete_node_by_value(head, value):
current = head
prev = None
while current and current.data != value:
prev = current
current = current.next
if current is not None:
prev.next = current.next
else:
print(f"Value {value} not found in the list.")
return head
```
阅读全文
相关推荐
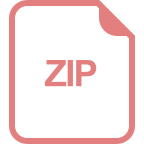
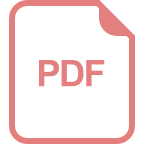

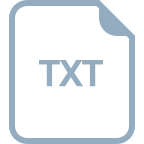
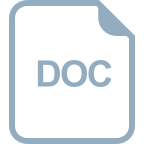


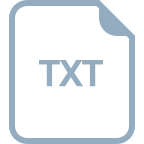
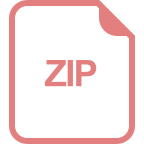
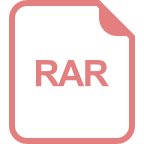
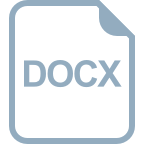
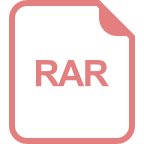
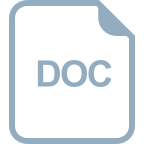




