python的继承类
时间: 2024-10-29 16:03:03 浏览: 15
在Python中,继承是一种创建新类的方式,它允许一个新类(称为子类或派生类)从另一个已存在的类(称为父类或超类)继承所有或部分属性和方法。通过继承,子类能够复用父类的结构,并且可以添加或修改自己的特性。
基本语法如下:
```python
class 子类名(父类名):
# 自己的属性和方法
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs) # 调用父类的构造函数
# 其他初始化代码
# 子类特有的方法
def method_name(self):
# 方法体
```
例如,如果有一个`Animal`类,我们可以创建一个`Dog`子类,继承其通用的行为:
```python
class Animal:
def sound(self):
print("动物的声音")
class Dog(Animal):
def sound(self): # 重写父类方法
print("汪汪")
dog = Dog()
dog.sound() # 输出 "汪汪"
```
相关问题
python继承类方法
在Python中,继承类方法可以通过在子类中定义同名的方法来实现。子类可以继承父类的方法并且可以重写父类的方法。当子类调用这个方法时,会优先调用子类中的方法。
下面是一个简单的示例代码,演示了如何在子类中继承父类的方法:
```python
class ParentClass:
def method(self):
print("This is a method from the parent class.")
class ChildClass(ParentClass):
pass
obj = ChildClass()
obj.method()
```
输出结果为:
```
This is a method from the parent class.
```
在上面的示例中,`ParentClass` 是父类,而 `ChildClass` 是子类。子类 `ChildClass` 并没有定义自己的 `method` 方法,所以它继承了父类 `ParentClass` 中的 `method` 方法。
如果你想在子类中重写父类的方法,可以在子类中定义一个同名的方法,并在其中实现子类自己的逻辑。例如:
```python
class ParentClass:
def method(self):
print("This is a method from the parent class.")
class ChildClass(ParentClass):
def method(self):
print("This is a method from the child class.")
obj = ChildClass()
obj.method()
```
输出结果为:
```
This is a method from the child class.
```
在上面的示例中,子类 `ChildClass` 重写了父类 `ParentClass` 中的 `method` 方法,并实现了自己的逻辑。当子类对象调用 `method` 方法时,会优先调用子类中的方法。
python 继承类怎么使用
在 Python 中,继承是一个关键的概念,用于创建一个新的类(子类)基于已存在的类(父类)。这使得子类能够继承并扩展父类的属性和方法,而无需重新编写相同的代码。以下是使用继承的基本步骤:
1. **定义父类(Base Class)**:
首先,定义一个父类,通常包含一些通用的方法或属性。例如:
```python
class Animal:
def __init__(self, name):
self.name = name
def sound(self):
pass
```
这里 `Animal` 类有一个 `name` 属性和一个抽象方法 `sound()`。
2. **定义子类(Derived Class)**:
使用 `extends` 或 `:` 后跟父类名来创建子类,并可能覆盖或添加新功能:
```python
class Dog(Animal):
def sound(self):
return "汪"
class Cat(Animal):
def sound(self):
return "喵"
```
在这里,`Dog` 和 `Cat` 类继承了 `Animal` 类,并重写了 `sound()` 方法。
3. **实例化子类**:
可以像操作普通类一样创建子类的实例:
```python
dog = Dog("旺财")
cat = Cat("小花")
print(dog.sound()) # 输出: "汪"
print(cat.sound()) # 输出: "喵"
```
4. **访问父类属性**:
子类可以直接访问父类的所有非私有属性和方法:
```python
dog.name = "旺财"
print(dog.name) # 输出: "旺财"
```
阅读全文
相关推荐
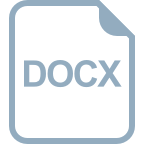
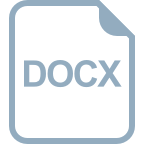
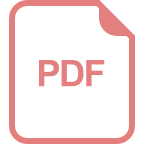













