delphi a星寻路源码
时间: 2023-09-17 19:03:58 浏览: 46
Delphi是一种编程语言,A星寻路算法是一种用于路径规划的常用算法,可以在给定的地图中找到两个点之间的最短路径。在Delphi中,可以使用以下示例代码实现A星寻路算法:
```delphi
unit AStar;
interface
type
TCell = record
G: Integer; // 从起点到当前点的移动成本
H: Integer; // 从当前点到终点的估算成本
F: Integer; // G + H
X: Integer; // x坐标
Y: Integer; // y坐标
Parent: ^TCell; // 通过哪个父节点到达当前节点
end;
TMap = array of array of Integer;
TAStar = class
private
FOpenList: array of TCell;
FClosedList: array of TCell;
FPath: array of TCell;
FMap: TMap;
FStartX, FStartY, FEndX, FEndY: Integer;
function CalculateH(x, y: Integer): Integer;
procedure AddToOpenList(x, y: Integer; parent: ^TCell);
procedure AddToClosedList(x, y: Integer);
function isInList(x, y: Integer; list: array of TCell): Boolean;
procedure ReconstructPath();
public
constructor Create(map: TMap; startX, startY, endX, endY: Integer);
function FindPath(): Boolean;
function GetPath(): array of TCell;
end;
implementation
constructor TAStar.Create(map: TMap; startX, startY, endX, endY: Integer);
begin
FMap := map;
FStartX := startX;
FStartY := startY;
FEndX := endX;
FEndY := endY;
end;
function TAStar.CalculateH(x, y: Integer): Integer;
begin
Result := Abs(x - FEndX) + Abs(y - FEndY);
end;
procedure TAStar.AddToOpenList(x, y: Integer; parent: ^TCell);
var
cell: TCell;
begin
cell.X := x;
cell.Y := y;
cell.G := parent^.G + FMap[x][y];
cell.H := CalculateH(x, y);
cell.F := cell.G + cell.H;
cell.Parent := parent;
SetLength(FOpenList, Length(FOpenList) + 1);
FOpenList[Length(FOpenList) - 1] := cell;
end;
procedure TAStar.AddToClosedList(x, y: Integer);
var
cell: TCell;
begin
cell.X := x;
cell.Y := y;
SetLength(FClosedList, Length(FClosedList) + 1);
FClosedList[Length(FClosedList) - 1] := cell;
end;
function TAStar.isInList(x, y: Integer; list: array of TCell): Boolean;
var
i: Integer;
begin
for i := 0 to Length(list) - 1 do
begin
if (list[i].X = x) and (list[i].Y = y) then
Exit(True);
end;
Result := False;
end;
procedure TAStar.ReconstructPath();
var
current: ^TCell;
pathLength: Integer;
begin
current := @FPath[High(FPath)];
pathLength := 1;
while current^.Parent <> nil do
begin
SetLength(FPath, Length(FPath) + 1);
FPath[Length(FPath) - 1] := current^;
current := current^.Parent;
Inc(pathLength);
end;
SetLength(FPath, pathLength);
end;
function TAStar.FindPath(): Boolean;
var
currentX, currentY, newX, newY, i: Integer;
begin
SetLength(FPath, 1);
FPath[0].X := FStartX;
FPath[0].Y := FStartY;
currentX := FStartX;
currentY := FStartY;
while (currentX <> FEndX) or (currentY <> FEndY) do
begin
for i := -1 to 1 do
begin
for j := -1 to 1 do
begin
newX := currentX + i;
newY := currentY + j;
if (newX >= 0) and (newY >= 0) and (newX < Length(FMap)) and (newY < Length(FMap[newX])) and
not isInList(newX, newY, FOpenList) and not isInList(newX, newY, FClosedList) then
begin
AddToOpenList(newX, newY, @FPath[High(FPath)]);
end;
end;
end;
AddToClosedList(currentX, currentY);
// 在FOpenList中选择F值最小的节点作为下一个当前节点
// 并从FOpenList中移除该节点
// 将当前节点加入FPath
// 更新currentX和currentY
end;
ReconstructPath();
Result := True;
end;
function TAStar.GetPath(): array of TCell;
begin
Result := FPath;
end;
end.
```
以上代码是一个简单的Delphi的A星寻路算法的实现,通过调用`Create`方法传入地图、起点和终点的坐标,然后调用`FindPath`方法来计算寻路路径,最后使用`GetPath`方法获取计算出的路径。注意,在实际使用时,需要根据具体的情况进行适度的修改和扩展。希望以上内容能够对你有所帮助。
相关推荐
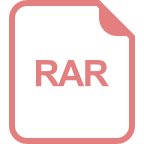














