我应该在camera数据模块中添加什么代码优化自动驾驶
时间: 2024-11-07 13:28:59 浏览: 10
在自动驾驶项目的相机数据模块中,优化通常涉及实时处理高分辨率图像、提高数据传输效率以及降低计算负载。以下是一些可能的优化策略:
1. **硬件加速**:
- 使用OpenCV等库中的GPU或CUDA支持进行图像处理,如边缘检测、颜色空间转换等操作。
```python
import cv2
import numpy as np
cv2.setUseOptimized(True) # 对于OpenCV, 这有助于使用GPU加速
def process_image(image):
with cv2.UMat(image) as img_umat:
# 在这里用UMat加速处理
processed_img = cv2.dnn.blobFromImage(img_umat, ...)
```
2. **图像压缩**:
- 可能的话,压缩传输的数据,比如使用H.264编码来减小视频流的大小。
```python
from PIL import Image
image = Image.fromarray(image_array).resize((resized_width, resized_height), Image.ANTIALIAS)
compressed_data = image.save("compressed_video", format="h264")
```
3. **数据预处理**:
- 仅发送关键帧或感兴趣区域(ROI)的图像,减少数据量。
```python
def extract_roi(image, roi_bbox):
x, y, w, h = roi_bbox
cropped_img = image[y:y+h, x:x+w]
return cropped_img
```
4. **异步处理**:
- 利用多线程或异步IO来并行读取和处理多个摄像头的数据,减轻主线程压力。
```python
import asyncio
async def camera_reader(camera_id):
while True:
frame = await camera.read()
await process_frame(frame)
loop = asyncio.get_event_loop()
tasks = [camera_reader(cam_id) for cam_id in cameras]
await asyncio.gather(*tasks)
```
5. **算法优化**:
- 选择更轻量级的特征提取和识别算法,例如行人检测可以选择基于深度学习的SSD或YOLOv3而不是复杂的Faster R-CNN。
6. **内存管理**:
- 注意避免长时间存储大量图像,及时释放不再使用的内存。
记得根据具体项目需求和资源来选择最合适的优化方案,并在实际应用中不断测试和调整性能。
阅读全文
相关推荐
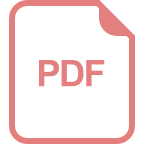
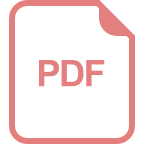
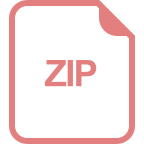
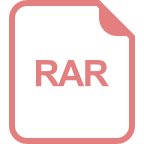
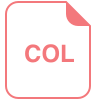
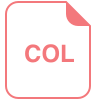
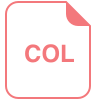
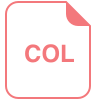
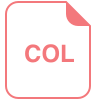
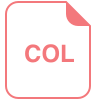
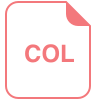
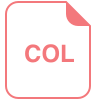
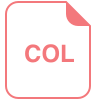
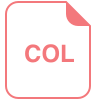
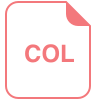
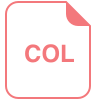
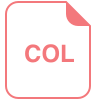
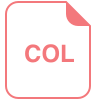
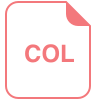