如何用C++编写一个会跳动的爱心图案程序?
时间: 2024-10-21 20:01:20 浏览: 51
在C++中创建一个会跳动的爱心图案可以使用控制台动画或者图形库,如ncurses或SFML。这里我将提供一种简单的控制台动画示例,它会让爱心每秒跳动一次:
```cpp
#include <iostream>
#include <chrono> // 引入时间库
using namespace std;
// 简单的心形字符数组
const char heart[] = {'/', '\\', '|', '/', '\\'};
void print_heart(int x, int y) {
cout << setw(4) << setw(y - 1) << setfill(' ') << "|";
for (int i = 0; i <= y; ++i) {
cout << setw(x) << heart[i % 5];
}
cout << endl;
}
void jump_heart(int x, int y, int speed) {
for (int i = 0; i <= y; ++i) {
for (int j = 0; j <= speed; ++j) {
print_heart(x + j, i);
this_thread::sleep_for(std::chrono::milliseconds(100)); // 暂停一段时间模拟跳动效果
}
}
}
int main() {
int x = 3; // 爱心初始位置
int y = 5; // 爱心的高度
int speed = 2; // 跳跃速度
while (true) { // 无限循环,让爱心一直跳动
jump_heart(x, y, speed);
x++; // 爱心每次跳动后向右移动
if (x > 80) { // 如果超出屏幕宽度,则重置到左边
x = 0;
}
}
return 0;
}
```
这个程序会在屏幕上不断显示一个向上跳动的心形,注意实际运行时可能会因为终端大小限制而无法完全展示,你可以调整`x`、`y`和`speed`值。
阅读全文
相关推荐
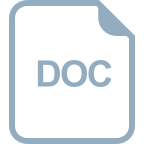
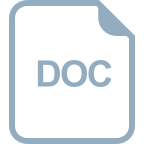
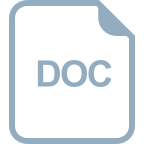








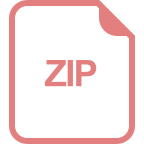
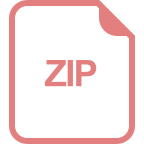
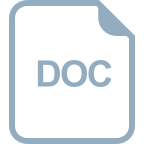




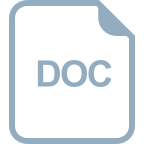