请列举出让图片批量打乱重命名的代码
时间: 2024-09-08 20:03:50 浏览: 22
要实现图片批量打乱重命名的功能,可以使用Python编程语言,并结合`os`和`random`模块来完成。以下是一个简单的代码示例,它将指定目录下的所有图片文件打乱顺序后重命名:
```python
import os
import random
import shutil
# 指定包含图片的文件夹路径
folder_path = 'path/to/your/folder'
# 指定新的文件名前缀
new_name_prefix = 'new_image_'
# 获取文件夹内所有文件的列表
files = os.listdir(folder_path)
# 过滤出图片文件(假设图片文件的扩展名为.jpg)
image_files = [f for f in files if f.endswith('.jpg')]
# 生成一个随机数列表,与图片文件列表长度相同
random_numbers = [random.randint(1, 100000) for _ in image_files]
# 打乱图片文件列表的顺序
random.shuffle(image_files)
# 遍历打乱后的图片文件列表,并进行重命名
for idx, image_file in enumerate(image_files):
# 分离文件名和扩展名
name_without_ext, ext = os.path.splitext(image_file)
# 构造新的文件名
new_name = f"{new_name_prefix}{random_numbers[idx]}{ext}"
# 构造旧文件和新文件的完整路径
old_file = os.path.join(folder_path, image_file)
new_file = os.path.join(folder_path, new_name)
# 重命名文件
os.rename(old_file, new_file)
print("图片批量打乱重命名完成!")
```
这段代码首先获取指定文件夹内的所有图片文件,然后打乱它们的顺序,并使用随机生成的数字作为新的文件名进行重命名。请注意,你需要根据实际情况修改`folder_path`和`new_name_prefix`变量的值,以及图片文件的扩展名。
相关推荐
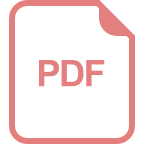
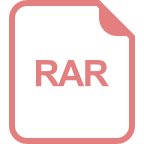
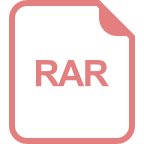














