C#中如何发送短信验证码?
时间: 2024-12-09 22:28:30 浏览: 16
在C#中,直接发送短信验证码通常涉及到第三方服务提供商,因为这超出了标准库的功能范围。下面是一个简化的示例,说明如何使用一个假设的外部API来发送短信验证码:
```csharp
using System.Net.Http;
using Newtonsoft.Json;
public async Task SendSmsCode(string phoneNumber, string code)
{
// 确保已设置好有效的短信服务API配置
const string apiKey = "your_api_key";
const string apiUrl = "https://api.smsprovider.com/send?phone={phoneNumber}&code={code}";
try
{
using (var client = new HttpClient())
{
client.DefaultRequestHeaders.Authorization =
new AuthenticationHeaderValue("Bearer", apiKey);
var content = new StringContent($"phone={phoneNumber}&code={code}", Encoding.UTF8, "application/x-www-form-urlencoded");
var response = await client.PostAsync(apiUrl, content);
if (!response.IsSuccessStatusCode)
{
throw new Exception($"Failed to send SMS, status code: {response.StatusCode}");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error sending SMS: {ex.Message}");
}
}
// 调用该方法传入手机号和验证码
await SendSmsCode(phoneNumber, GenerateRandomCode());
```
在这个例子中,你需要替换`your_api_key`和`apiUrl`为你实际使用的短信服务提供商的API密钥和URL,并且`GenerateRandomCode()`函数用于生成随机验证码。
请注意,上述代码仅为演示目的,真实情况下的短信发送通常会更复杂,包括身份验证、错误处理以及可能的延迟等。
阅读全文
相关推荐








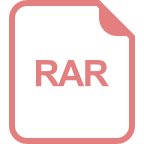



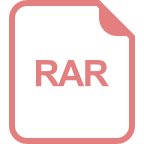





