os.open PermissionError: [Errno 13] Permission denied:
时间: 2024-02-18 18:58:09 浏览: 38
os.open是Python中的一个函数,用于打开文件并返回文件描述符。它的语法如下:
```python
os.open(file, flags[, mode])
```
其中,file是要打开的文件名,flags是打开文件的模式,mode是可选参数,用于设置文件的权限。
当你尝试使用os.open打开一个文件时,可能会遇到PermissionError: [Errno 13] Permission denied错误。这是因为你没有足够的权限来打开该文件。
这个错误通常发生在以下情况下:
- 你尝试打开一个只读文件,但是你没有读取该文件的权限。
- 你尝试打开一个只写文件,但是你没有写入该文件的权限。
- 你尝试打开一个目录而不是文件。
要解决这个错误,你可以尝试以下几种方法:
1. 检查文件或目录的权限,确保你具有读取或写入的权限。
2. 如果你是在Linux或Mac系统上运行Python程序,可以尝试使用sudo命令以管理员身份运行程序,这样可以获得更高的权限。
3. 如果你是在Windows系统上运行Python程序,可以尝试右键点击程序并选择“以管理员身份运行”。
希望以上信息对你有帮助!
相关问题
python中PermissionError: [Errno 13] Permission denied:
在Python中,当您尝试打开或写入文件时,如果当前用户没有足够的权限来修改或读取文件,就会出现"PermissionError: [Errno 13] Permission denied"错误。这是一个常见的文件权限问题。
要解决这个问题,您可以使用os模块中的chmod函数来更改文件的权限。但在尝试更改文件权限之前,请确保您拥有足够的权限来修改文件。
以下是一个示例代码,演示如何处理"PermissionError: [Errno 13] Permission denied"错误:
```python
import os
try:
# 尝试打开或写入文件
with open('file.txt', 'w') as file:
file.write('Hello, World!')
except PermissionError:
# 如果出现权限错误,尝试更改文件权限
os.chmod('file.txt', 0o777)
# 再次尝试打开或写入文件
with open('file.txt', 'w') as file:
file.write('Hello, World!')
```
请注意,上述代码中的'file.txt'是一个示例文件名,您需要将其替换为您实际使用的文件名。
PermissionError: [Errno 13] Permission denied: 'output.xlsx'
"PermissionError: [Errno 13] Permission denied: 'output.xlsx'"是一个Python中的错误消息,表示在尝试打开或写入文件时没有足够的权限。根据引用、和中的描述,这个错误通常发生在以下几种情况下:
1. 文件正在被其他程序占用或正在被另一个Python脚本打开。如果在使用openpyxl或pandas库时遇到这个错误,可以尝试确保在打开或写入文件之前将其关闭。可以使用`close()`方法来关闭文件。可以通过使用`with`语句来确保文件被正确关闭,例如:
```
with open('output.xlsx', 'w') as file:
# 执行文件操作
```
2. 文件所在的目录没有写入权限。在某些情况下,可能是由于目录的权限设置导致无法写入文件。确保你有足够的权限来在指定的目录中创建或修改文件。可以使用操作系统的文件管理工具来检查和更改目录权限。
3. 文件已被其他进程或用户锁定。如果其他进程或用户正在使用该文件,你可能无法在Python中访问或修改它。在这种情况下,你可以等待其他进程或用户释放文件锁定,或者联系其他进程或用户关闭文件。
总结来说,"PermissionError: [Errno 13] Permission denied: 'output.xlsx'"错误通常是由于文件被其他程序占用、目录权限设置不正确或文件被锁定而引起的。你可以通过关闭文件、检查和更改目录权限或等待其他进程或用户释放文件锁定来解决这个问题。
相关推荐
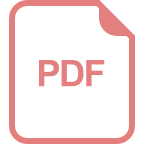
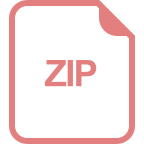
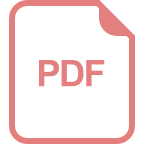












