如何在Apache HttpClient 4.5.13版本中实现GET请求并附带HTTP body(POST数据)?
时间: 2024-12-19 19:27:29 浏览: 9
在Apache HttpClient 4.5.13版本中,GET请求通常不携带HTTP body(POST数据),因为GET用于获取资源,而POST用于提交数据。但是,如果你想模拟一个带数据的GET请求(尽管这在HTTP标准中并不常见),你可以创建一个`HttpGet`对象,并设置它的uri,然后将其与`HttpEntity`结合,如`UrlEncodedFormEntity`。
以下是简单的示例:
```java
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public CloseableHttpResponse sendGetWithBody(String baseUrl, Map<String, String> params) throws Exception {
HttpGet request = new HttpGet(baseUrl);
// 将POST数据编码为URL查询字符串
StringBuilder urlBuilder = new StringBuilder(baseUrl);
if (params != null && !params.isEmpty()) {
urlBuilder.append("?");
for (Map.Entry<String, String> entry : params.entrySet()) {
urlBuilder.append(entry.getKey()).append("=").append(URLEncoder.encode(entry.getValue(), "UTF-8")).append("&");
}
urlBuilder.setLength(urlBuilder.length() - 1); // 移除最后一个 &
}
request.setURI(new URI(urlBuilder.toString()));
// 创建一个StringEntity来包含我们想要发送的数据
StringEntity entity = new StringEntity(urlBuilder.toString(), ContentType.APPLICATION_FORM_URLENCODED);
// 设置实体到请求中
request.setEntity(entity);
CloseableHttpClient httpClient = HttpClients.createDefault();
try {
return httpClient.execute(request);
} finally {
httpClient.close();
}
}
// 使用示例
Map<String, String> params = new HashMap<>();
params.put("param1", "value1");
params.put("param2", "value2");
CloseableHttpResponse response = sendGetWithBody("http://example.com/api", params);
try {
System.out.println(EntityUtils.toString(response.getEntity()));
} finally {
response.close();
}
```
阅读全文
相关推荐
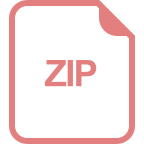
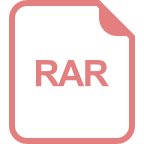
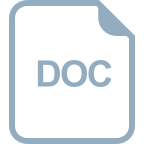
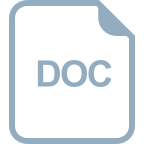
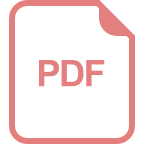
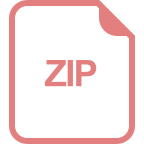
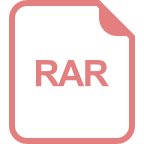
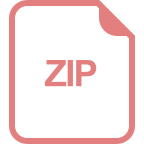
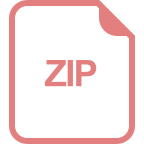
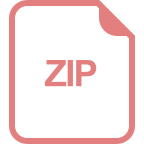
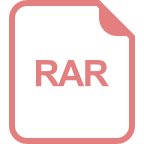
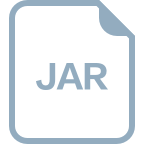
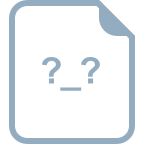