python操纵数据库
时间: 2024-10-15 13:04:17 浏览: 22
在Python中,有许多库用于操作数据库,其中最流行的是SQLAlchemy和SQLite。以下是关于Python数据库操作的一些基本信息:
1. **SQLite**: SQLite是一个轻量级的关系型数据库,内置在Python标准库中。你可以通过`sqlite3`模块直接与SQLite数据库交互,无需安装额外软件。
```python
import sqlite3
# 连接到SQLite数据库
conn = sqlite3.connect('example.db')
c = conn.cursor()
# 创建表
c.execute('''CREATE TABLE IF NOT EXISTS users
(id INTEGER PRIMARY KEY, name TEXT)''')
# 插入数据
c.execute("INSERT INTO users VALUES (1, 'Alice')")
conn.commit()
# 查询数据
cursor_result = c.execute("SELECT * FROM users WHERE id=1")
for row in cursor_result:
print(row)
# 关闭连接
conn.close()
```
2. **SQLAlchemy**: SQLAlchemy是一个强大的ORM(对象关系映射)库,它提供了一种更高级的方式来处理数据库操作,可以与多种数据库系统如MySQL、PostgreSQL等无缝集成。
```python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
engine = create_engine('sqlite:///example.db') # 使用SQLite
Base.metadata.create_all(engine)
# 使用Session对象进行操作
session = Session(bind=engine)
new_user = User(name='Bob')
session.add(new_user)
session.commit()
```
阅读全文
相关推荐
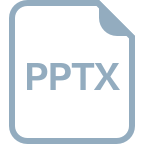
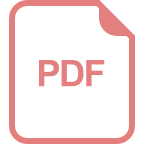
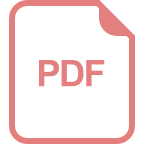
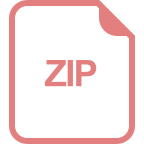
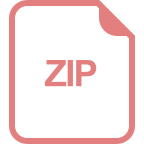
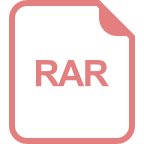
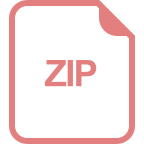
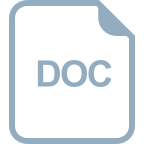
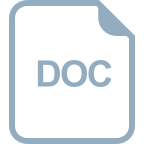
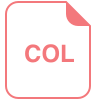
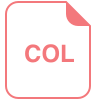


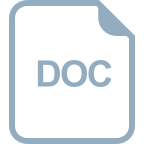
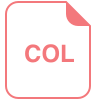
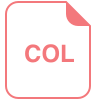
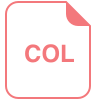
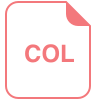
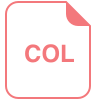