编写完整的C++语言程序完成下列实验任务: 自学头插法建立单链表 首先用头插法建立一个长度为10的带头结点的单链表,值任意;然后遍历此单链表,将10个元素的值在屏幕上输出。(简化起见,假设数据元素类型为int类型) 实现按位查找,并输出结果
时间: 2024-09-19 19:04:31 浏览: 42
在C++中,我们可以创建一个简单的单链表结构,包含节点类型和头结点。下面是一个基本的实现,包括头插法构建链表以及遍历并打印链表内容。同时,我们还会实现一个按位查找的函数。
```cpp
#include <iostream>
// 定义链表节点结构
struct Node {
int data;
Node* next;
};
// 创建一个新的链表节点
Node* createNode(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
return newNode;
}
// 头插法构建链表
Node* buildLinkedList(int length) {
Node* head = new Node(); // 创建头结点
for (int i = 0; i < length; ++i) {
Node* newNode = createNode(i + 1); // 从1开始计数
newNode->next = head->next; // 插入到链表头部
head->next = newNode;
}
return head;
}
// 遍历并打印链表
void printList(Node* head) {
Node* current = head->next; // 跳过头结点
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
// 按位查找
bool findBit(int target, int position, int listElement) {
return (listElement & (1 << position)) ? true : false;
}
// 主函数
int main() {
const int length = 10;
Node* head = buildLinkedList(length);
std::cout << "链表元素:";
printList(head);
int targetValue = 5; // 例如我们要查找的目标值
for (int i = 0; i <= sizeof(int) * 8; ++i) { // 8位二进制数
if (findBit(targetValue, i, head->data)) {
std::cout << "在第" << i+1 << "位找到!" << std::endl;
} else {
std::cout << "在第" << i+1 << "位未找到." << std::endl;
}
}
delete head; // 销毁链表
return 0;
}
```
这个程序首先创建了一个长度为10的链表,然后打印所有元素。接着,它会检查目标值5在每个位置上是否出现在链表的第一个元素中(假设所有元素都是整型)。`findBit`函数通过按位操作来判断目标值的某一位在链表元素中的存在情况。
阅读全文
相关推荐
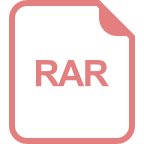
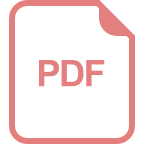
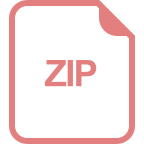


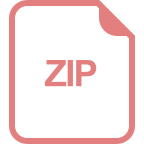
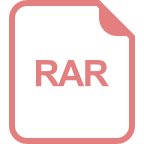
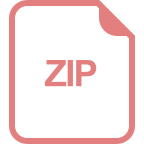
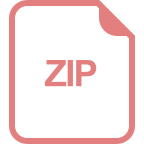
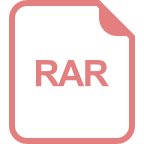
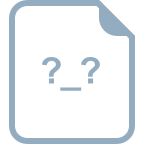
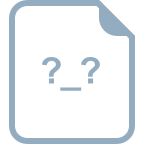
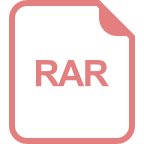
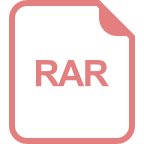
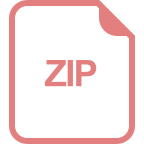
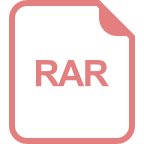
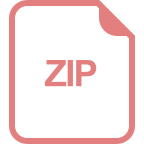