java导入excel表到数据库
时间: 2023-11-29 09:42:19 浏览: 55
可以使用Apache POI库来读取Excel文件,然后使用JDBC连接数据库,将数据插入到数据库中。以下是一个简单的示例代码:
```java
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToDatabase {
public static void main(String[] args) {
String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
String username = "root";
String password = "password";
String excelFilePath = "data.xlsx";
try (Connection connection = DriverManager.getConnection(jdbcUrl, username, password);
FileInputStream inputStream = new FileInputStream(excelFilePath);
Workbook workbook = new XSSFWorkbook(inputStream)) {
Sheet sheet = workbook.getSheetAt(0);
String sql = "INSERT INTO mytable (column1, column2, column3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
int rowNumber = 0;
for (Row row : sheet) {
if (rowNumber == 0) {
rowNumber++;
continue;
}
Cell cell1 = row.getCell(0);
String column1 = cell1.getStringCellValue();
Cell cell2 = row.getCell(1);
int column2 = (int) cell2.getNumericCellValue();
Cell cell3 = row.getCell(2);
double column3 = cell3.getNumericCellValue();
statement.setString(1, column1);
statement.setInt(2, column2);
statement.setDouble(3, column3);
statement.addBatch();
if (rowNumber % 100 == 0) {
statement.executeBatch();
}
}
workbook.close();
statement.executeBatch();
System.out.println("Data imported successfully.");
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
相关推荐
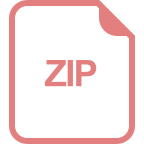














