安卓activity页面中布局有textview ,button recycleView 这些控件如何能够响应遥控器的上下左右移动到对应的控件上并确定键实现示例java demo
时间: 2024-09-18 21:05:55 浏览: 15
在Android中,为了使Activity页面中的TextView、Button和RecyclerView等控件能响应遥控器的方向键操作,你可以创建一个`OnKeyDownEventListener`来监听键盘事件,并根据方向键值进行相应的处理。下面是一个简单的Java示例:
首先,在你的Activity中设置一个全局变量,用于存储当前焦点所在的控件:
```java
private View currentFocus;
```
然后,添加一个初始化方法,设置默认焦点:
```java
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化焦点为第一个可见的控件
View firstVisibleView = findViewById(android.R.id.content).getRootView().findViewById(findViewById(R.id.main_layout).getFirstChildAt(0));
if (firstVisibleView != null) {
currentFocus = firstVisibleView;
currentFocus.requestFocus();
}
}
```
接着,实现`OnKeyDownEventListener`:
```java
public class RemoteControlHandling extends AppCompatActivity implements View.OnKeyListener {
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
switch (keyCode) {
case KeyEvent.KEYCODE_DPAD_UP: // 上箭头
handleUpDirection(v);
break;
case KeyEvent.KEYCODE_DPAD_DOWN: // 下箭头
handleDownDirection(v);
break;
case KeyEvent.KEYCODE_DPAD_LEFT: // 左箭头
handleLeftDirection(v);
break;
case KeyEvent.KEYCODE_DPAD_RIGHT: // 右箭头
handleRightDirection(v);
break;
// 其他方向键处理...
default:
return false; // 如果不是我们需要的方向键,返回false,让系统处理其他事件
}
return true; // 回应了键盘事件
}
private void handleUpDirection(View view) {
if (currentFocus == view) {
// 遍历焦点之前的控件
View previousFocus = findPreviousFocus(view);
if (previousFocus != null) {
previousFocus.requestFocus();
currentFocus = previousFocus;
} else {
// 如果已到达顶部,焦点回到底部
RecyclerView recyclerView = findViewById(R.id.recyclerView);
if (recyclerView != null && recyclerView.getAdapter() != null) {
recyclerView.smoothScrollToPosition(recyclerView.getAdapter().getItemCount() - 1);
currentFocus = recyclerView.getChildAt(recyclerView.getAdapter().getItemCount() - 1);
} else {
currentFocus = findViewById(R.id.button);
}
}
}
}
// ...其他方向处理函数类似,只是改变焦点的逻辑
private View findPreviousFocus(View startView) {
if (startView instanceof ViewGroup) {
ViewGroup parent = (ViewGroup) startView;
for (int i = parent.getChildCount() - 1; i >= 0; i--) {
View child = parent.getChildAt(i);
if (child.equals(currentFocus)) {
continue;
}
if (child.isFocusable() && child.isFocused()) {
return child;
}
}
}
return null;
}
}
```
在这个例子中,`handleUpDirection`、`handleDownDirection`、`handleLeftDirection` 和 `handleRightDirection` 分别处理四个方向键,通过遍历控件或RecyclerView的滚动来找到下一个焦点。
相关推荐
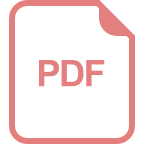
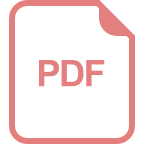
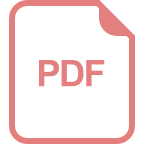














