使用go语言写一个post请求
时间: 2024-10-03 16:01:23 浏览: 28
在Go语言中,你可以使用`net/http`包来发送HTTP POST请求。下面是一个简单的例子:
```go
package main
import (
"bytes"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
type Data struct {
Key string `json:"key"`
Value string `json:"value"`
}
func main() {
url := "http://example.com/api" // 替换为你实际的API地址
data := Data{Key: "your_key", Value: "your_value"}
jsonData, err := json.Marshal(data)
if err != nil {
fmt.Println("Error marshaling data:", err)
return
}
requestBody := bytes.NewBuffer(jsonData)
req, err := http.NewRequest("POST", url, requestBody)
if err != nil {
fmt.Println("Error creating request:", err)
return
}
req.Header.Set("Content-Type", "application/json") // 设置请求头,指定内容类型
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error sending request:", err)
} else {
defer resp.Body.Close()
body, _ := ioutil.ReadAll(resp.Body) // 读取响应体
fmt.Println(string(body))
if resp.StatusCode == http.StatusOK { // 检查HTTP状态码
fmt.Println("Post successful")
} else {
fmt.Printf("Post failed with status code %d\n", resp.StatusCode)
}
}
}
```
在这个示例里,我们首先创建了一个JSON格式的数据结构,然后将其转换为字节并设置为POST请求的主体。记得替换`url`、`data`以及错误处理部分的内容以适应实际需求。
阅读全文
相关推荐
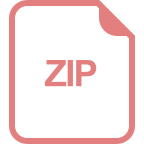
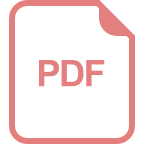
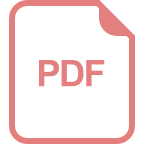
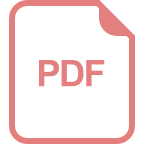
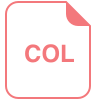





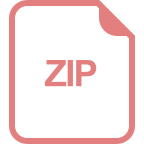
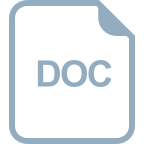





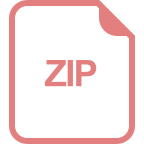