AES encryption algorithm should be used with secured mode
时间: 2024-09-14 13:13:42 浏览: 66
AES (Advanced Encryption Standard) is a widely adopted symmetric-key cryptographic algorithm, which stands for Advanced Encryption Standard. It's highly secure and efficient for encrypting data in Java. When using AES for encryption, it's crucial to employ a secure mode of operation to ensure the confidentiality and integrity of your data.
In Java, the `javax.crypto.Cipher` class provides various modes of operation for symmetric ciphers, including AES. Here are some secure modes you should consider:
1. **AES/CBC (Cipher Block Chaining)**: This mode is often preferred because it adds an initialization vector (IV) before each block of plaintext. CBC ensures that each block depends on the previous one, making it more resistant to known-plaintext attacks. You'll need to securely manage the IV to maintain secrecy.
2. **AES/GCM (Galois/Counter Mode)**: GCM is a popular authenticated encryption mode that combines encryption with message authentication code (MAC). It offers both confidentiality and integrity protection in a single step, making it a good choice when you want to prevent tampering.
3. **AES/CTR (Counter mode)**: CTR also provides confidentiality but without built-in integrity checking like GCM. However, it's suitable if you plan to apply separate MAC or hash functions for authenticity.
To use AES with a secure mode in Java, follow these steps:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
// Generate a random secret key and initialization vector
SecretKeySpec key = new SecretKeySpec(secretKey.getBytes(StandardCharsets.UTF_8), "AES");
IvParameterSpec iv = new IvParameterSpec(randomIV.getBytes(StandardCharsets.UTF_8));
// Create a Cipher instance and set the mode (CBC or GCM)
Cipher cipher;
if (mode == Cipher.ENCRYPT_MODE) {
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); // For CBC
} else if (mode == Cipher.DECRYPT_MODE) {
cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); // Same for decryption
// If using GCM, use Cipher.getInstance("AES/GCM/NoPadding")
}
// Initialize the cipher with the key and IV
cipher.init(mode, key, iv);
byte[] encryptedData = cipher.doFinal(plaintext);
```
Remember to keep the key and IV secure, as they're critical for decryption. The IV should be randomly generated and not reused for multiple encryptions.
阅读全文
相关推荐
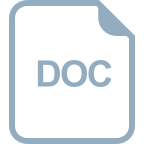
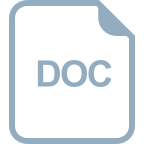
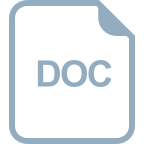
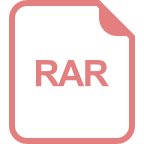
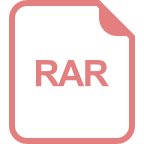
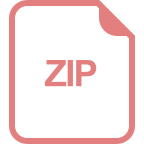
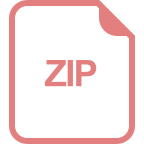
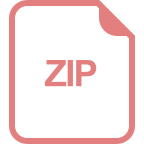
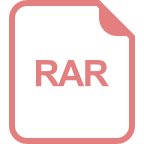
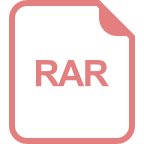
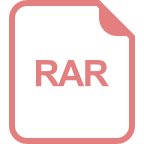
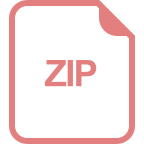
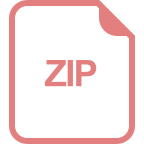
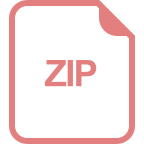
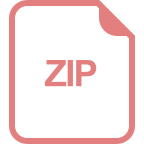
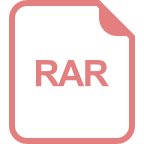