用c代码实现S-AES的加密解密过程
时间: 2024-10-16 21:12:47 浏览: 38
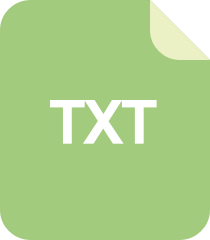
java代码-java使用AES加密解密 AES-128-ECB加密
AES (Advanced Encryption Standard) 是一种广泛使用的块密码算法,用于对数据进行安全加密。在C语言中,使用OpenSSL库可以方便地实现AES的加密和解密操作。以下是使用OpenSSL库基本步骤:
```c
#include <openssl/aes.h>
#include <openssl/evp.h>
#include <string.h>
// AES Key size and block size in bytes
const int key_size = AES_KEY_SIZE; // For AES-128, AES-192 or AES-256
const int block_size = AES_BLOCK_SIZE;
void encrypt(char *plaintext, char *key, char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key((unsigned char*)key, key_size * 8, &aes_key);
unsigned char iv[AES_BLOCK_SIZE]; // Initialize with zeros if not using a specific IV
memset(iv, 0, AES_BLOCK_SIZE);
// Create an encryption context with the key and initialization vector
EVP_CIPHER_CTX evp_cipher_ctx;
EVP_CipherInit(&evp_cipher_ctx, EVP_aes_128_cbc(), NULL, NULL, iv);
// Encrypt data
int len = strlen(plaintext);
ciphertext = malloc(len + AES_BLOCK_SIZE);
if (!EVP_CipherUpdate(&evp_cipher_ctx, ciphertext, &len, (unsigned char*)plaintext, len)) {
printf("Encryption error\n");
free(ciphertext);
return;
}
// Add padding and finalize the encryption
ciphertext[len] = AES_BLOCK_SIZE - (len % AES_BLOCK_SIZE); // Add padding byte(s)
if (!EVP_CipherFinal(&evp_cipher_ctx, ciphertext + len, &len)) {
printf("Encryption error\n");
}
ciphertext[len] = '\0'; // Terminate the ciphertext string
// Store any necessary information for decryption, such as IV
}
void decrypt(char *ciphertext, char *key, char *plaintext) {
AES_KEY aes_key;
AES_set_decrypt_key((unsigned char*)key, key_size * 8, &aes_key);
unsigned char iv[AES_BLOCK_SIZE];
// You would typically retrieve the IV from somewhere here, depending on your application
// Reuse the same encryption context but switch to decryption mode
EVP_CIPHER_CTX_init(&evp_cipher_ctx);
EVP_CipherInit(&evp_cipher_ctx, EVP_aes_128_cbc(), NULL, &iv, NULL);
int len = strlen(ciphertext);
plaintext = malloc(len);
if (!EVP_CipherUpdate(&evp_cipher_ctx, plaintext, &len, ciphertext, len)) {
printf("Decryption error\n");
free(plaintext);
return;
}
// Remove padding
int padding = ciphertext[len - 1];
for (int i = len - 1; i >= 0 && plaintext[i] == padding; i--) {}
plaintext[i+1] = '\0';
if (!EVP_CipherFinal(&evp_cipher_ctx, plaintext + len, &len)) {
printf("Decryption error\n");
}
free(ciphertext); // Free memory allocated for ciphertext
}
阅读全文
相关推荐
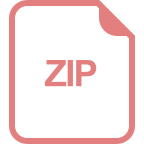
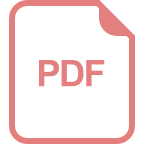















