编程从键盘输入某年某月(包括闰年),用switch语句编程输出该年的该月拥有的天数。要求考虑闰年以及输入月份不在合法范围内的情况。已知闰年的2月有29天,平年的2月有28天
时间: 2024-10-22 09:13:12 浏览: 25
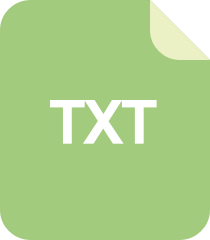
编程实现从键盘上输入年份和月份,即可输出该页对应的天数含(闰年
在编程中,你可以通过使用`switch`语句结合条件判断来实现这个功能。首先,你需要获取用户输入的年份和月份,然后检查它是否属于合法范围(1到12)。接着,你可以编写一个针对各个月份的天数规则:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入年份:");
int year = scanner.nextInt();
System.out.println("请输入月份(1-12):");
int month = scanner.nextInt();
// 检查输入合法性
if (month < 1 || month > 12) {
System.out.println("月份输入错误,只能选择1到12个月份.");
return;
}
switch (month) {
case 1: // January
case 3: // March
case 5: // May
case 7: // July
case 8: // August
case 10: // October
case 12: // December
System.out.println(year + "年的" + month + "月共有31天.");
break;
case 4: // April
case 6: // June
case 9: // September
case 11: // November
System.out.println(year + "年的" + month + "月共有30天.");
break;
case 2: // February
if (isLeapYear(year)) { // 判断是否为闰年
System.out.println(year + "年的2月共有29天.");
} else {
System.out.println(year + "年的2月共有28天.");
}
break;
default:
System.out.println("出错了,无法识别的月份.");
break;
}
scanner.close();
}
// 判断是否为闰年
private static boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
}
```
当运行此程序时,它会提示用户输入年份和月份,然后根据用户输入计算并输出相应月份的天数。如果输入的月份超出范围或不是常规月份(如非2月的二月),程序会给出相应的错误消息。
阅读全文
相关推荐
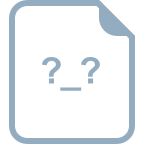















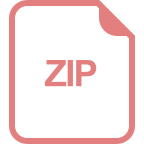