Open the data file using pandas and push data in numpy.array for plotting hydraulic fracturing operation diagram using matplotlib. You should obtain 4 figures something like.
时间: 2024-04-17 22:25:03 浏览: 123
Sure! To open the data file using pandas and plot the hydraulic fracturing operation diagram using matplotlib, you can follow the steps below:
1. Install the required libraries if you haven't already:
```python
pip install pandas matplotlib
```
2. Import the necessary libraries:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
```
3. Load the data file using pandas:
```python
data = pd.read_excel('path/to/data_file.xlsx')
```
Replace `'path/to/data_file.xlsx'` with the actual path to your data file.
4. Extract the necessary columns from the data:
Assuming your data file contains columns named `'Stage'`, `'Time'`, `'Pressure'`, and `'Rate'`, you can extract them as follows:
```python
stage = data['Stage']
time = data['Time']
pressure = data['Pressure']
rate = data['Rate']
```
5. Plot the hydraulic fracturing operation diagram:
```python
fig, axs = plt.subplots(2, 2, figsize=(12, 8))
axs[0, 0].plot(stage, pressure)
axs[0, 0].set_xlabel('Stage')
axs[0, 0].set_ylabel('Pressure')
axs[0, 1].plot(stage, rate)
axs[0, 1].set_xlabel('Stage')
axs[0, 1].set_ylabel('Rate')
axs[1, 0].plot(time, pressure)
axs[1, 0].set_xlabel('Time')
axs[1, 0].set_ylabel('Pressure')
axs[1, 1].plot(time, rate)
axs[1, 1].set_xlabel('Time')
axs[1, 1].set_ylabel('Rate')
plt.tight_layout()
plt.show()
```
The above code will create a 2x2 grid of subplots, where each subplot represents a different combination of `'Stage'`, `'Time'`, `'Pressure'`, and `'Rate'`. Adjust the size of the figure (`figsize`) as needed.
Make sure to replace `'Stage'`, `'Time'`, `'Pressure'`, and `'Rate'` with the actual column names in your data file.
Hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐











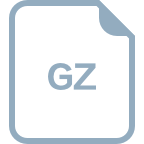
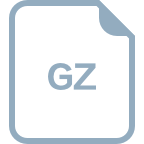
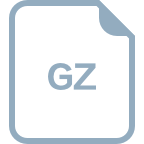
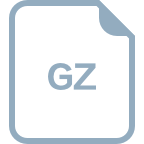
