编写程序,对一副彩色图像进行灰度化、二值化、均值化操作。 2. 编写程序,对图像进行4邻域连通区域标记 (connected component labeling, CCL) fRiF. 输入图像1 输入图像2:连通区域标记
时间: 2024-09-30 11:06:44 浏览: 169
1. 对于彩色图像的处理,首先需要将其转换为灰度图像。在Python中,可以使用PIL(Pillow库)来进行这个操作。例如:
```python
from PIL import Image
def grayscale_image(image_path):
with Image.open(image_path) as img:
gray_img = img.convert('L')
return gray_img
```
接下来,为了进行二值化,你可以选择基于阈值的处理,比如Otsu's thresholding算法,或者自定义的阈值设置:
```python
from skimage import exposure
def binary_threshold(gray_img, threshold_method='otsu'):
if threshold_method == 'otsu':
binary_img = gray_img > exposure.otsu(gray_img)
else:
# 自定义阈值
binary_img = gray_img > 100 # 假设100是一个合适的阈值
return binary_img
```
对于均值化,通常是平滑图像的一种方式,可以用PIL的filter函数实现平均滤波:
```python
def mean_filter(binary_img, kernel_size=3):
blurred_img = binary_img.filter(ImageFilter.MedianFilter(kernel_size))
return blurred_img
```
2. 进行4邻域连通区域标记(Connected Component Labeling, CCL),可以使用OpenCV的`cv2.connectedComponentsWithStats`函数。这里给出一个简单的示例:
```python
import cv2
import numpy as np
def ccl(image_path):
img = cv2.imread(image_path, 0) # 读取灰度图像
labeled_img, num_labels = cv2.connectedComponents(img)
# 可能会添加区域统计信息,如面积、中心等
stats, centroids = cv2.connectedComponentsWithStats(labeled_img)
return labeled_img, num_labels, stats, centroids
```
对于输入的两个图像,你需要分别对它们进行上述步骤。如果你想要将这两个过程合并到一个文件中,可以考虑创建函数组合:
```python
def process_images(image1_path, image2_path):
gray1 = grayscale_image(image1_path)
binary1 = binary_threshold(gray1)
mean1 = mean_filter(binary1)
labeled1, _, _, _ = ccl(mean1)
# 同样的步骤对image2进行处理...
gray2, binary2, mean2, labeled2 = ...
# 返回结果
```
阅读全文
相关推荐
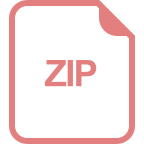
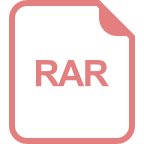
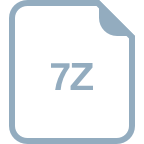

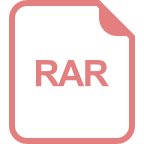
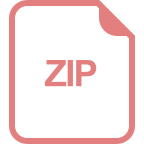
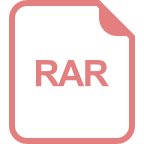
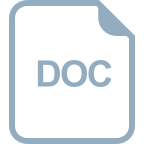
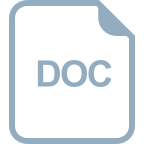
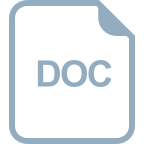
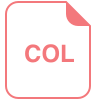
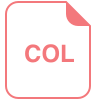
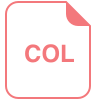
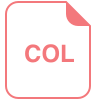
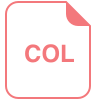
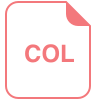
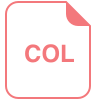
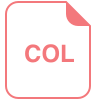
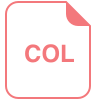
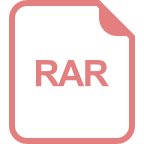