python爬虫写一个爬取天气信息并制成图表的代码
时间: 2024-10-26 21:03:43 浏览: 19
在Python中编写一个简单的天气信息爬虫并制作成图表,通常需要几个步骤:
1. **选择合适的API或网站**:首先,你需要找到提供天气数据的API(如OpenWeatherMap、AccuWeather等),或者直接访问一些公开的天气网站,比如中国气象局的API。
2. **安装必要的库**:安装`requests`库用于发送HTTP请求获取数据,以及`pandas`处理数据,`matplotlib`用于生成图表。可以使用`pip install requests pandas matplotlib`命令来安装。
```bash
pip install requests pandas matplotlib
```
3. **编写爬虫函数**:
```python
import requests
import json
import pandas as pd
def get_weather_data(city):
api_key = "your_api_key" # 替换为你获取的API密钥
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
if response.status_code == 200:
data = json.loads(response.text)
return data['main']
else:
print(f"Error fetching data for {city}. Status code: {response.status_code}")
return None
def parse_weather_data(data):
temperature = data['temp'] - 273.15 # 转换为摄氏度
humidity = data['humidity']
return {'temperature': temperature, 'humidity': humidity}
def plot_weather_info(weather_data):
df = pd.DataFrame([weather_data])
df.plot(kind='bar', rot=0) # 绘制柱状图
plt.title('Temperature and Humidity')
plt.xlabel('Parameters')
plt.ylabel('Values')
plt.show()
city = input("请输入你想查询的城市名: ")
weather_data = get_weather_data(city)
if weather_data is not None:
weather_info = parse_weather_data(weather_data)
plot_weather_info(weather_info)
else:
print("无法获取数据")
```
4. **运行程序并查看结果**:输入你要查询的城市名,程序会抓取天气数据并生成气温和湿度的柱状图。
注意:这个示例假设你已经有一个可用的OpenWeatherMap API密钥。实际操作时,你可能需要注册并获取自己的API密钥,并遵守相应的服务条款。
阅读全文
相关推荐
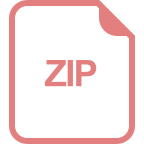
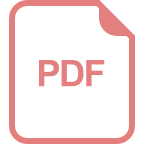
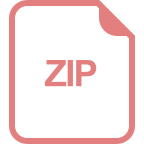
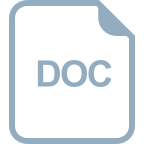
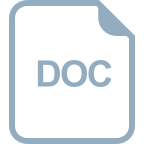
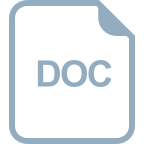
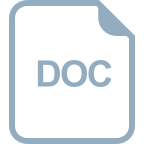
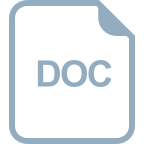
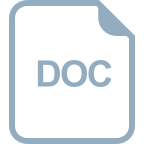
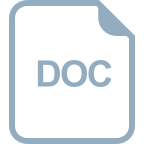
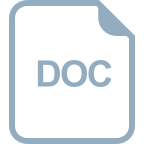
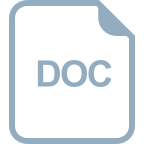
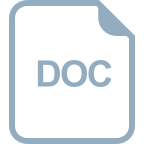
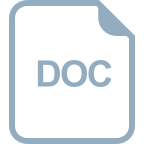
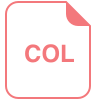



