C语言输入两个字符串,以回车隔开,母串在前,子串在后。计算子串出现的次数,并输出。 (母串大小不超过50,子串小于母串) 举例: 输入:abca a 输出:2 (子串'a'在母串‘abca’中出现次数为2) 注意:不允许直接调用库函数进行子串比较
时间: 2024-10-28 17:10:52 浏览: 19
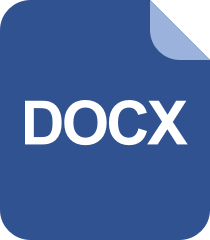
《常见字符串处理函数》.docx
在C语言中,你可以通过手动遍历字符串的方式来实现这个功能。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
int count_substring(char *str1, char *str2) {
int len1 = strlen(str1);
int len2 = strlen(str2);
int i, j;
int count = 0;
for (i = 0; i <= len1 - len2; i++) {
for (j = 0; j < len2; j++) {
if (str1[i + j] != str2[j]) {
break;
}
}
if (j == len2) { // 如果所有字符都匹配,则计数加一
count++;
}
}
return count;
}
int main() {
char mother[51], child[26]; // 假设输入字符串长度不大于50,子串小于50
printf("请输入母串(回车结束): ");
fgets(mother, sizeof(mother), stdin); // 获取用户输入并去除末尾换行符
mother[strlen(mother)-1] = '\0'; // 修正因fgets添加的换行符
printf("请输入子串: ");
fgets(child, sizeof(child), stdin);
child[strlen(child)-1] = '\0';
int result = count_substring(mother, child);
printf("子串 '%s' 在母串 '%s' 中出现的次数: %d\n", child, mother, result);
return 0;
}
```
在这个程序中,`count_substring` 函数接收两个字符串作为参数,然后使用嵌套循环逐个字符对比。如果找到完全匹配的子串,就增加计数器。主函数部分获取用户的输入,并调用这个函数。
注意:这个解决方案假设输入不会包含特殊字符,如空格或其他控制字符,仅适用于纯文本环境。如果你需要处理更复杂的情况,可能需要修改输入部分,例如添加错误检查和清理过程。
阅读全文
相关推荐
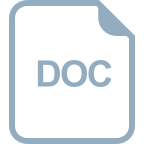
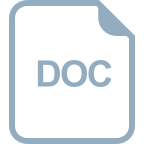

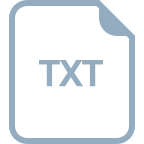
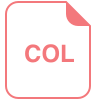












