graphsage pytorch训练完整代码
时间: 2023-08-31 20:02:34 浏览: 63
GraphSAGE(Graph Sample and Aggregated)是一种用于图神经网络的模型,可以对节点进行嵌入表示学习。下面是一个使用PyTorch实现GraphSAGE训练的完整代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.nn.parameter import Parameter
from torch_geometric.nn import SAGEConv
class GraphSAGE(nn.Module):
def __init__(self, num_nodes, embed_dim, num_neighbors):
super(GraphSAGE, self).__init__()
self.conv1 = SAGEConv(embed_dim, embed_dim)
self.conv2 = SAGEConv(embed_dim, embed_dim)
self.fc = nn.Linear(embed_dim, num_nodes)
self.num_neighbors = num_neighbors
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, p=0.5, training=self.training)
x = self.conv2(x, edge_index)
x = F.relu(x)
x = F.dropout(x, p=0.5, training=self.training)
x = self.fc(x)
return F.log_softmax(x, dim=1)
# 设置超参数
num_nodes = 100
embed_dim = 16
num_neighbors = 5
lr = 0.01
num_epochs = 10
# 创建模型实例
model = GraphSAGE(num_nodes, embed_dim, num_neighbors)
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=lr)
# 训练模型
for epoch in range(num_epochs):
optimizer.zero_grad()
output = model(x, edge_index)
loss = criterion(output, y)
loss.backward()
optimizer.step()
# 打印训练信息
if (epoch+1) % 5 == 0:
_, predicted = torch.max(output, 1)
correct = (predicted == y).sum().item()
accuracy = correct / len(y)
print(f'Epoch {epoch+1}/{num_epochs}, Loss: {loss.item()}, Accuracy: {accuracy}')
```
上面代码中,首先我们定义了一个GraphSAGE模型类,它包含了两个SAGEConv层和一个线性层。在forward函数中,我们通过多次调用SAGEConv层来对节点进行嵌入表示学习,最后使用线性层对嵌入表示进行分类。
然后我们定义了超参数,包括节点数量、嵌入维度、邻居数量、学习率和训练轮数。
接下来创建了模型实例,并定义了损失函数和优化器。
在训练过程中,我们循环迭代多个epoch,每个epoch中进行前向传播、计算损失、反向传播和参数更新。在每个epoch的末尾,我们计算并打印损失和准确率。
以上就是使用PyTorch实现GraphSAGE训练的完整代码。请注意,上述代码假设已经准备好了节点特征x和边索引edge_index,以及对应的真实标签y。
相关推荐
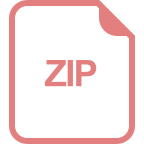
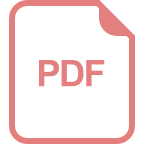














