帮我写一个测试指令的程序,能测RISC架构RV32IMAC指令集的全部指令
时间: 2024-11-27 14:13:02 浏览: 7
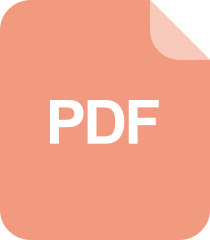
实验课指令集.pdf
编写一个针对RISC-V RV32IMAC架构指令集的全面测试程序需要考虑以下几个步骤。首先,你需要了解RV32IMAC指令集的组成,包括基础运算、算术操作、加载/存储以及控制转移等。RISC-V指令通常由固定长度的字节表示,并且有明确的操作码字段。
下面是一个简化版的Python示例,展示如何创建一个基本的测试框架。请注意这只是一个简化的例子,实际的测试框架可能会更复杂,涉及断言、循环和输入数据生成等:
```python
import unittest
from riscv_test_generator import RV32IMACInstructionSet
class TestRV32IMACInstructions(unittest.TestCase):
def setUp(self):
self.instruction_set = RV32IMACInstructionSet()
def test_arithmetic_instructions(self):
# 测试加法、减法、乘法和移位指令
for instr_name in self.instruction_set.arithmetic_instructions:
instr = getattr(self.instruction_set, instr_name)
self.assertIsNotNone(instr.execute(), f"Arithmetic instruction {instr_name} failed.")
def test_load_store_instructions(self):
# 测试LW, SW等加载/存储指令
for instr_name in self.instruction_set.load_store_instructions:
instr = getattr(self.instruction_set, instr_name)
self.assertEqual(True, instr.is_supported(), f"Load/store instruction {instr_name} not supported.")
def test_branch_instructions(self):
# 测试B, JAL等分支指令
for instr_name in self.instruction_set.branch_instructions:
instr = getattr(self.instruction_set, instr_name)
# 这里假设所有分支都成功跳转,真正的测试可能需要检查目标地址
self.assertEqual(True, instr.execute() is None, f"Branch instruction {instr_name} failed.")
def tearDown(self):
pass
if __name__ == '__main__':
unittest.main()
```
在这个程序中,`riscv_test_generator.RV32IMACInstructionSet`是一个假想的库,包含RV32IMAC指令的集合和相关的执行函数。你需要自定义这个库以匹配RV32IMAC的具体细节。
阅读全文
相关推荐
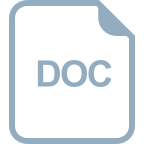
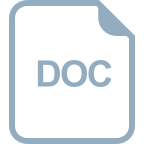
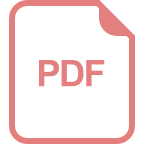
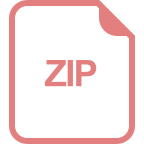
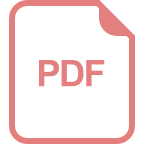
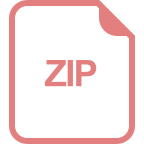
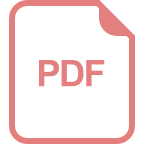
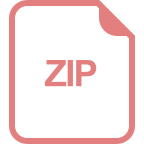
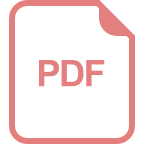
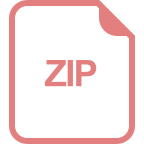
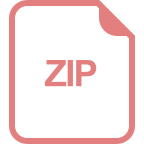
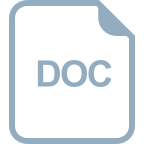
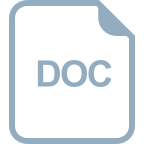



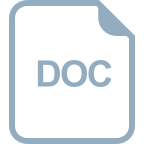
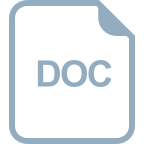