springboot怎么发送邮箱验证码
时间: 2023-07-21 11:06:15 浏览: 52
在 Spring Boot 中发送邮箱验证码可以通过使用 JavaMailSender 来实现。首先,你需要在 `application.properties` 或 `application.yml` 配置文件中配置邮件服务器的相关信息,如下所示:
application.properties:
```properties
spring.mail.host=your.mail.server
spring.mail.port=your.mail.port
spring.mail.username=your.username
spring.mail.password=your.password
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
```
application.yml:
```yaml
spring:
mail:
host: your.mail.server
port: your.mail.port
username: your.username
password: your.password
properties:
mail:
smtp:
auth: true
starttls:
enable: true
```
接下来,你可以创建一个邮件服务类来发送验证邮件,示例如下:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
@Autowired
private JavaMailSender javaMailSender;
public void sendVerificationCode(String to, String code) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject("验证码");
message.setText("您的验证码是:" + code);
javaMailSender.send(message);
}
}
```
在上述示例中,`JavaMailSender` 是由 Spring Boot 自动配置的邮件发送器。你可以在需要发送验证码的地方调用 `sendVerificationCode` 方法来发送邮件。
注意:为了使用JavaMailSender,你需要在项目的依赖管理文件(例如 pom.xml)中添加相应的依赖。你可以添加以下依赖来使用 Spring Boot 提供的邮件支持:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
```
以上就是使用 Spring Boot 发送邮箱验证码的简单示例。你可以根据自己的实际需求进行调整和扩展。
相关推荐
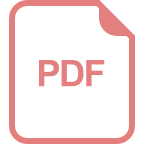
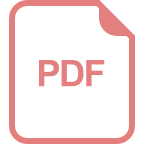














