python中的指针详解
时间: 2023-10-23 13:00:19 浏览: 184
在Python中,没有直接的指针概念。相反,Python中的变量是对对象的引用。这意味着变量存储了对象在内存中的地址,而不是对象本身。
当你创建一个对象并将其赋值给一个变量时,变量实际上是指向该对象的引用。如果你将一个变量赋值给另一个变量,那么两个变量都将引用同一个对象。这就是为什么在Python中可以通过一个变量修改另一个变量所引用的对象。
例如,考虑以下代码:
```
a = [1, 2, 3]
b = a
```
在这个例子中,变量`a`引用了一个包含整数1、2和3的列表对象。然后,我们将`a`赋值给变量`b`,这意味着`b`也引用同一个列表对象。
现在,如果我们修改`a`所引用的列表对象,`b`也会反映这些更改:
```
a.append(4)
print(b) # 输出结果为 [1, 2, 3, 4]
```
由于`a`和`b`都指向同一个列表对象,所以对`a`的更改会影响到`b`。
需要注意的是,在Python中,整数、字符串和元组等不可变类型的对象是不能被修改的。当你试图修改不可变对象时,实际上是创建了一个新的对象,并将变量指向该新对象。
虽然Python中没有显式的指针概念,但理解变量是对对象的引用可以帮助你更好地理解Python中的变量和对象之间的关系。
相关问题
python中链表详解
链表是一种常见的数据结构,用于存储一系列的元素。相比于数组,链表的插入和删除操作更加高效。在Python中,可以使用类来实现链表。
一个链表由一个个节点组成,每个节点包含两个部分:数据和指向下一个节点的指针。
下面是一个简单的链表的示例:
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if self.head is None:
self.head = new_node
else:
current = self.head
while current.next is not None:
current = current.next
current.next = new_node
def insert(self, data, position):
if position < 0 or position > self.length():
raise ValueError("Invalid position")
new_node = Node(data)
if position == 0:
new_node.next = self.head
self.head = new_node
else:
current = self.head
for _ in range(position - 1):
current = current.next
new_node.next = current.next
current.next = new_node
def remove(self, data):
if self.head is None:
raise ValueError("LinkedList is empty")
if self.head.data == data:
self.head = self.head.next
else:
current = self.head
while current.next is not None:
if current.next.data == data:
current.next = current.next.next
return
current = current.next
raise ValueError("Data not found")
def length(self):
count = 0
current = self.head
while current is not None:
count += 1
current = current.next
return count
def print_list(self):
current = self.head
while current is not None:
print(current.data, end=" ")
current = current.next
print()
```
上述代码中,`Node`类表示链表中的节点,包含`data`和`next`两个属性。`LinkedList`类表示链表,包含`head`属性作为链表的头节点。
`append`方法用于向链表末尾添加一个节点。如果链表为空,新节点将成为头节点;否则,遍历链表直到找到末尾节点,将新节点添加在其后。
`insert`方法用于在指定位置插入一个节点。如果位置为0,新节点将成为头节点;否则,遍历链表到达指定位置,将新节点插入在当前节点之后。
`remove`方法用于删除链表中的某个节点。如果要删除的节点是头节点,将头节点指向下一个节点;否则,遍历链表直到找到要删除的节点,将当前节点的`next`指针指向要删除节点的下一个节点。
`length`方法用于计算链表的长度。
`print_list`方法用于打印链表中的所有元素。
可以使用以下代码创建一个链表并进行操作:
```python
# 创建链表
my_list = LinkedList()
# 添加元素
my_list.append(1)
my_list.append(2)
my_list.append(3)
# 插入元素
my_list.insert(4, 1)
# 打印链表
my_list.print_list() # 输出: 1 4 2 3
# 删除元素
my_list.remove(2)
# 打印链表
my_list.print_list() # 输出: 1 4 3
# 计算链表长度
print(my_list.length()) # 输出: 3
```
这是链表的基本实现,你可以根据需要进行扩展和修改。
python 文件指针
在Python中,文件指针是用来标记文件中读写位置的一个概念。文件指针指向文件中的某个位置,可以根据需要进行读取或写入操作。对于读取操作,文件指针会随着读取的字节数向后移动;对于写入操作,文件指针会随着写入的字节数向后移动。
在刷新缓冲区之前,需要关闭文件以确保写入的信息被保存。在关闭文件后,文件指针将不再可用,无法进行读写操作。
在Python中,读写操作共用一个文件指针,即读取操作和写入操作使用的是同一个文件指针。这意味着,在进行写入操作之前,如果文件指针已经移动到了文件末尾,那么读取操作将无法读取到任何内容。
在文件的读写操作中,需要注意文件指针之间的相互影响。例如,在读取操作后,文件指针会向后移动读取的字节数;在写入操作后,文件指针也会向后移动写入的字节数。因此,在进行文件操作时,需要谨慎处理文件指针的位置。
另外,Python提供了一些方法来控制文件指针的位置。例如,可以使用seek()方法将文件指针移动到指定位置,使用tell()方法获取当前文件指针的位置。
truncate()方法用于将文件截断到指定的长度。在使用truncate()方法后,文件指针的位置不会受到影响,仍然指向截断后的位置。
综上所述,Python中的文件指针是用来标记文件中读写位置的概念。在读写操作中,文件指针会根据读写的字节数向后移动。需要注意文件指针之间的相互影响,并可以使用seek()和tell()等方法来控制文件指针的位置。同时,truncate()方法可以用于截断文件并保留文件指针的位置。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [python 文件指针详解、文件基本操作方法及在文件起始位置插入内容](https://blog.csdn.net/qq_42779673/article/details/122950316)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [python读写文件——文件指针操作](https://blog.csdn.net/Arider/article/details/117821424)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐


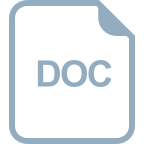










