用java的MapReduce写一个完整的一定能够跑通的MapReduce程序,目的是将这个文档中的数据以行为单位分割后,将每行的倒数第二列数据类型拆分成2017-11-23这种的类型后与改行的其他数据一起保存下来,最后检查每一行数据中是否有多余的空格,如果有就删除
时间: 2024-09-22 11:01:26 浏览: 38
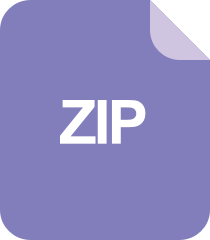
Hadoop的分割程序的例子。程序显示我们如何在Hadoop的MapReduce程序使用一个分区.zip
为了实现您所描述的需求,我们可以设计一个简单的Java MapReduce程序来处理文本文件中的每一行记录,并对日期格式进行调整以及去除多余的空格。下面是一个基本的框架示例:
### Mapper类
Mapper的主要任务是从输入的文本行中读取数据,并将每行按照逗号分隔符切分,然后转换日期格式并清理多余空格。
```java
import java.io.IOException;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class DateFormatterMapper extends Mapper<LongWritable, Text, Text, Text> {
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] fields = line.split(",");
// 检查是否有足够的字段
if (fields.length >= 2) {
// 转换日期格式: YYYYMMDD -> YYYY-MM-DD
String dateStr = fields[fields.length - 2];
String formattedDate = String.format("%s-%s-%s", dateStr.substring(0, 4), dateStr.substring(4, 6), dateStr.substring(6));
// 清理多余的空格
for (int i = 0; i < fields.length; i++) {
fields[i] = fields[i].trim();
}
// 将格式化后的日期放回原位置
fields[fields.length - 2] = formattedDate;
// 构建新的字符串输出
String outputLine = String.join(",", fields);
context.write(new Text(outputLine), new Text("processed"));
}
}
}
```
### Reducer类
Reducer在这里主要用于收集和确认Mapper阶段的结果,这里可以简单地将结果直接写出。
```java
import java.io.IOException;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class DateFormatterReducer extends Reducer<Text, Text, Text, NullWritable> {
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text val : values) {
context.write(key, NullWritable.get());
}
}
}
```
### 主函数
在主函数中配置Job参数,设置输入输出路径等。
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.NullWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class DateFormatterDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "date_formatter");
job.setJarByClass(DateFormatterDriver.class);
job.setMapperClass(DateFormatterMapper.class);
job.setReducerClass(DateFormatterReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(NullWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码提供了一个基础的MapReduce流程来处理您的需求,请根据实际情况调整输入输出路径以及其他细节部分。注意运行此程序需要Hadoop环境支持。
阅读全文
相关推荐
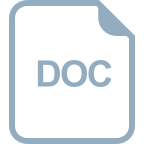
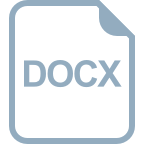
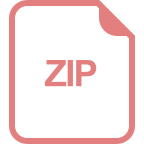
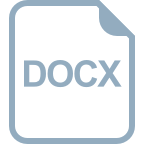
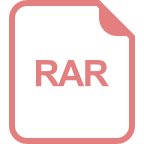
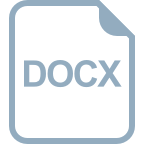
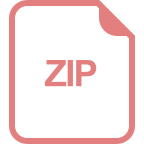
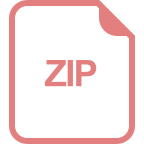
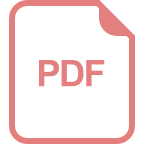
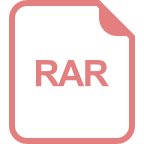
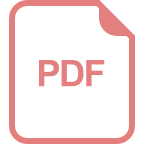
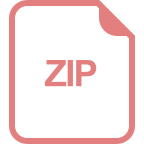
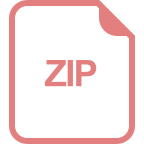
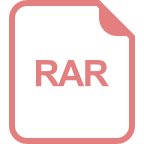
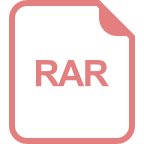
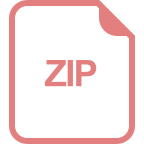