AttributeError: 'NoneType' object has no attribute 'xy'
时间: 2024-12-26 07:21:25 浏览: 4
### 解决 Python 中 `AttributeError: 'NoneType' object has no attribute` 错误
当遇到 `'NoneType' object has no attribute` 的错误时,通常意味着尝试访问的对象为 `None` 而不是一个有效的实例。这可能发生在多种场景下,比如对象未被正确初始化、返回值为空或是外部资源加载失败。
#### 检查并验证对象的有效性
在执行任何属性调用之前,应该先确认目标对象确实存在且不为 `None`。可以通过简单的条件判断来实现这一点:
```python
if obj is not None and hasattr(obj, "attribute"):
value = getattr(obj, "attribute")
else:
print("Object does not exist or lacks the specified attribute.")
```
对于特定情况下的处理方式如下:
#### 文件读取问题
如果是在文件操作过程中遇到了此异常,则需确保文件路径正确无误,并且文件能够成功打开。例如,在使用 OpenCV 库读取图像时,应当检查图片是否真的被加载到了内存中[^4]:
```python
import cv2
image_path = "path/to/your/image.jpg"
img = cv2.imread(image_path)
if img is not None:
# 继续后续逻辑...
else:
print(f"Failed to load image from {image_path}")
```
#### Web Scraping 场景
如果是网络爬虫项目里发生的此类错误,特别是涉及 HTML 文档解析的时候,要留意网页结构的变化可能导致某些元素找不到的情况。此时可考虑增加对页面响应状态码的检测以及更稳健的选择器策略[^2]:
```python
from lxml import html
import requests
url = "http://example.com/"
response = requests.get(url)
if response.status_code == 200:
tree = html.fromstring(response.content)
elements = tree.xpath("//div[@class='target']")
if elements:
element = elements[0]
text_content = element.text_content()
# 进一步的操作...
else:
print("Target element was not found on page.")
else:
print(f"Request failed with status code {response.status_code}.")
```
通过以上措施可以在很大程度上减少因意外获取到 `None` 值而引发的相关错误。同时建议开发人员养成良好的编程习惯,提前预防潜在的风险点。
阅读全文
相关推荐
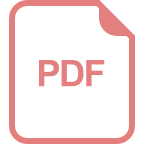
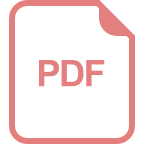
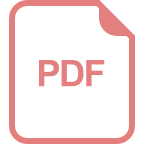















