帮我写出上述代码的完整代码
时间: 2024-06-23 14:01:09 浏览: 148
在C++中,我们可以使用模板和继承等概念来创建一个多态的类。这里是一个简单的例子,展示了一个基类`Shape`及其派生类`Circle`和`Rectangle`,以及一个函数`printArea()`使用了多态:
```cpp
#include <iostream>
// 基类 Shape
class Shape {
public:
virtual double getArea() const = 0; // 纯虚函数,声明不提供实现
};
// 派生类 Circle
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() const override { return 3.14 * radius * radius; }
};
// 派生类 Rectangle
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double getArea() const override { return width * height; }
};
// 函数 printArea() 利用多态
void printArea(const Shape& shape) {
std::cout << "Area of the shape: " << shape.getArea() << std::endl;
}
int main() {
Shape* circlePtr = new Circle(5);
Shape* rectanglePtr = new Rectangle(4, 6);
printArea(*circlePtr); // 输出 Circle 的面积
printArea(*rectanglePtr); // 输出 Rectangle 的面积
delete circlePtr;
delete rectanglePtr;
return 0;
}
阅读全文
相关推荐

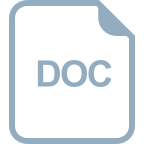
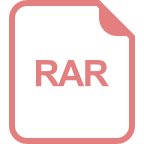








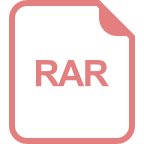

