python提取图片中颜色占比
时间: 2024-12-16 14:18:16 浏览: 1
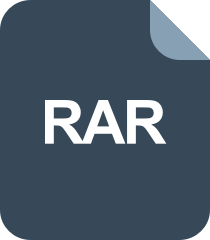
README_hee77_python文字提取_用python提取图片中文字_图片文字提取_

在Python中,你可以使用`PIL`库来处理图像,并结合`sklearn.cluster`模块的KMeans算法来提取主要颜色。这里有一个基本示例说明如何执行这个任务[^1]:
```python
from PIL import Image
import numpy as np
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
def extract_colors(image_path, n_colors=5):
# 1. 加载图像并转换为RGB
img = Image.open(image_path)
img_array = np.array(img.convert('RGB'))
# 2. 转换为二维数组并应用KMeans
flat_img = img_array.reshape(-1, 3)
kmeans = KMeans(n_clusters=n_colors)
colors = kmeans.fit_predict(flat_img)
# 3. 计算颜色占比和像素数
color_counts = {color: np.sum(colors == i) for i, color in enumerate(kmeans.cluster_centers_)}
total_pixels = img_array.shape[0] * img_array.shape[1]
# 4. 创建颜色占比和像素统计的数据结构
color_stats = [(k, (v / total_pixels) * 100) for k, v in color_counts.items()]
# 5. 可视化结果
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.pie(color_counts.values(), labels=kmeans.cluster_centers_.astype(str), autopct='%1.1f%%')
plt.title('Color Pie Chart')
plt.subplot(1, 2, 2)
plt.bar(range(n_colors), color_counts.values(), tick_label=[str(i) for i in range(n_colors)])
plt.title('Bar Chart of Color Pixel Counts')
return color_stats
# 示例
image_path = "path_to_your_image.jpg"
colors_and_percentages = extract_colors(image_path)
```
要按照颜色占比对颜色进行排序[^2],可以在最后一步添加:
```python
sorted_color_stats = sorted(color_stats, key=lambda x: x[1], reverse=True)
```
这会返回一个列表,其中的颜色按占比降序排列。
阅读全文
相关推荐
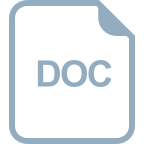
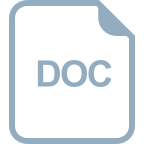


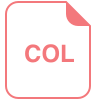
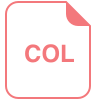
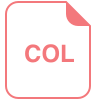
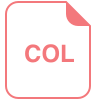
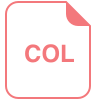
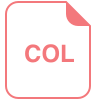



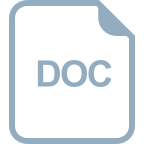
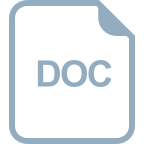
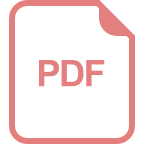
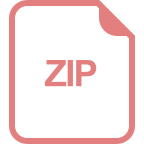