RabbitMQ如何实现延迟队列?
时间: 2023-10-30 11:55:52 浏览: 120
RabbitMQ可以通过插件的方式实现延迟队列。以下是一种常用的方法:
1. 首先,确保你的RabbitMQ服务器已经安装了rabbitmq_delayed_message_exchange插件。如果没有安装,你需要先安装该插件。
2. 创建一个延迟队列,你可以使用如下代码声明一个延迟队列:
```python
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
args = {
'x-delayed-type': 'direct',
}
channel.exchange_declare(exchange='delayed_exchange', exchange_type='x-delayed-message', arguments=args)
channel.queue_declare(queue='delayed_queue')
channel.queue_bind(queue='delayed_queue', exchange='delayed_exchange', routing_key='routing_key')
```
3. 发送延迟消息到延迟队列,你可以使用如下代码发送延迟消息:
```python
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
properties = pika.BasicProperties(headers={'x-delay': 5000}) # 设置延迟时间,单位为毫秒
channel.basic_publish(
exchange='delayed_exchange',
routing_key='routing_key',
body='Hello, delayed message!',
properties=properties
)
```
4. 消费延迟队列中的消息,你可以使用如下代码消费延迟消息:
```python
import pika
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
def callback(ch, method, properties, body):
print("Received delayed message:", body)
channel.basic_consume(queue='delayed_queue', on_message_callback=callback, auto_ack=True)
channel.start_consuming()
```
以上是通过插件实现延迟队列的方法,你可以根据自己的需求进行调整和扩展。
阅读全文
相关推荐
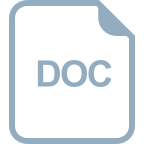
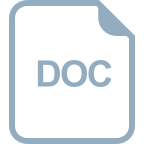
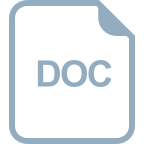
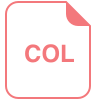


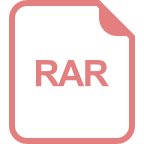
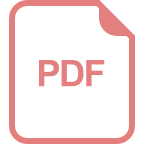
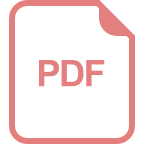
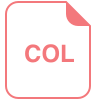
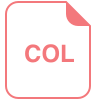







