面向对象程序设计考勤打卡系统
时间: 2024-12-26 16:18:38 浏览: 9
### 面向对象程序设计实现考勤打卡系统
#### 设计思路
在构建考勤打卡系统时,采用面向对象编程(OOP)能够有效提升代码的可维护性和扩展性。通过定义不同的类来表示实体及其行为,可以使整个应用程序结构更加清晰合理。
#### 类的设计
对于一个基本的考勤打卡系统来说,至少需要以下几个核心类:
- **User**: 用户基类,用于存储通用属性如用户名、密码等。
- **Student(User)** 和 **Teacher(User)** : 继承自`User`,分别代表学生和教师两种角色,各自拥有特定方法。
- **AttendanceRecord**: 记录每次签到的信息,包括时间戳、地点坐标(可通过高德地图API获取)、状态(正常/迟到/早退)[^1].
- **DatabaseHandler**: 处理与数据库交互的任务,负责保存新记录以及查询已有数据[^4].
下面给出一段简化版Python代码片段展示如何利用OOP原则创建这样一个简单的考勤管理工具:
```python
import datetime
class User:
def __init__(self, username, password):
self.username = username
self.password = password
@staticmethod
def login(users_data, input_username, input_password):
"""验证给定凭证"""
for user in users_data.values():
if (input_username == user['username'] and
input_password == user['password']):
return True
return False
class Student(User):
pass # 学生特有功能可以在子类中添加
class Teacher(User):
pass # 教师特有权限同样在此处定义
class AttendanceRecord:
def __init__(self, location_coords=None):
current_time = str(datetime.datetime.now())
# 假设location_coords由外部传入或调用某些地理信息服务获得
self.record_info = {
'timestamp': current_time,
'status': "present",
'coordinates': location_coords or {"lat": None,"lng":None}
}
def main():
sample_users = {'student_007':{'username':'s007','password':'pwd'}}
while True:
print("\n--- Welcome to the attendance system ---\n")
choice = input("[L]ogin | [Q]uit ? ").strip().lower()
if choice not in ['l', 'q']:
continue
elif choice == 'l':
name = input("Enter your Username:")
pwd = input("Password:")
is_validated = User.login(sample_users,name,pwd)
if is_validated:
new_record = AttendanceRecord({"lat":"39.9","lng":"116.4"})
print(f"\nWelcome {name}, you've successfully checked-in at Beijing.")
else:
print("Invalid credentials!")
else:
break
if __name__ == '__main__':
main()
```
这段代码展示了基础架构,实际应用还需要考虑更多细节,例如异常处理机制、更复杂的业务逻辑支持等等。
阅读全文
相关推荐







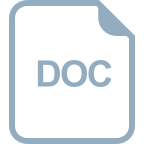


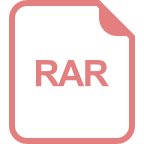






