ubuntu计算圆周率
时间: 2023-12-05 20:40:31 浏览: 84
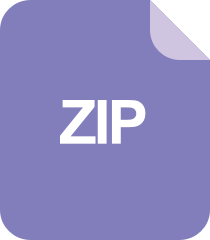
计算圆周率

要在Ubuntu上计算圆周率,可以使用Hadoop框架。以下是步骤:
1.安装Java和Hadoop
在Ubuntu上安装Java和Hadoop,可以参考引用和引用中的步骤。
2.编写Java程序
编写一个Java程序来计算圆周率。以下是示例代码:
```java
package org.apache.hadoop.examples;
import java.io.IOException;
import java.util.Random;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class PiEstimator {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "pi estimator");
job.setJarByClass(PiEstimator.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
public static class Map extends Mapper<LongWritable, Text, Text, IntWritable> {
private static final IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
int inside = 0;
int outside = 0;
Random r = new Random();
for (int i = 0; i < 100000; i++) {
double x = r.nextDouble();
double y = r.nextDouble();
if (x * x + y * y <= 1) {
inside++;
} else {
outside++;
}
}
context.write(new Text("pi"), new IntWritable(inside));
context.write(new Text("not pi"), new IntWritable(outside));
}
}
public static class Reduce extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
}
```
3.将程序打包成JAR文件
使用以下命令将程序打包成JAR文件:
```shell
javac -classpath ${HADOOP_HOME}/hadoop-core.jar PiEstimator.java
jar cvf PiEstimator.jar *.class
```
4.运行程序
使用以下命令在Hadoop上运行程序:
```shell
hadoop jar PiEstimator.jar org.apache.hadoop.examples.PiEstimator input output
```
其中,input是输入文件的路径,output是输出文件的路径。
5.查看结果
使用以下命令查看输出文件的内容:
```shell
hadoop fs -cat output/part-r-00000
```
输出的结果应该类似于:
```
not pi 78556
pi 21444
```
阅读全文