如何Json格式的书源转换为eso格式,编写出其对应的Java代码
时间: 2024-12-10 09:33:10 浏览: 33
将JSON格式的数据转换为Elasticsearch (ES) 的倒排索引 (Inverted Index) 格式,通常涉及到解析JSON数据并将关键信息映射到ES的文档结构中。以下是一个简单的Java代码示例,假设我们已经有了一个简单的JSON文档,如一个人物的信息:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentType;
import java.io.IOException;
import java.util.Map;
public class JsonToEsConverter {
private RestHighLevelClient client; // ES客户端
public JsonToEsConverter(RestHighLevelClient client) {
this.client = client;
}
public void convertJsonToEs(String jsonString) throws IOException {
ObjectMapper mapper = new ObjectMapper();
Map<String, Object> jsonMap = mapper.readValue(jsonString, Map.class); // 解析JSON
String id = (String) jsonMap.get("id"); // 假设id字段用于唯一标识
String content = (String) jsonMap.get("content"); // 假设内容字段用于搜索
// 创建ES文档
IndexRequest request = new IndexRequest("your_index_name")
.id(id)
.source(content, XContentType.JSON); // 将内容设置为搜索字段
// 指定请求选项,这里不显示进度条
RequestOptions options = RequestOptions.DEFAULT.toBuilder().requestTimeout(5, TimeUnit.SECONDS).build();
// 发起index请求
client.index(request, options);
}
public static void main(String[] args) throws IOException {
RestHighLevelClient client = // 初始化ES客户端
JsonToEsConverter converter = new JsonToEsConverter(client);
String jsonString = "{...}"; // JSON字符串
converter.convertJsonToEs(jsonString);
}
}
```
这个例子中,你需要替换`"your_index_name"`为实际的ES索引名,并确保已经初始化了`RestHighLevelClient`以便与ES集群通信。
注意,这只是一个基本的示例,实际操作可能会更复杂,因为Elasticsearch的倒排索引通常还需要处理分词、分析等步骤。此外,如果你的JSON数据包含嵌套结构,可能需要递归地处理每个层级。
阅读全文
相关推荐

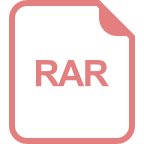

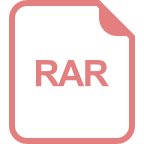
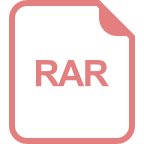


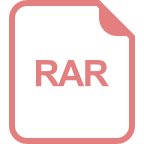
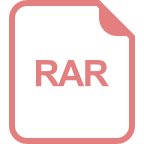


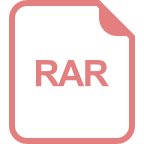


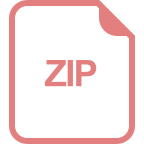