写一个C语言代码,利用数据结构链表实现集合的交并补
时间: 2024-09-27 12:05:38 浏览: 10
在C语言中,我们可以使用链表数据结构来实现集合的操作,比如交集、并集和补集。这里提供一个简单的示例,我们将使用`struct`来定义节点,然后分别创建函数来操作链表表示的集合。由于篇幅限制,我将展示基本的代码框架和部分关键函数,实际完整实现需要包含更多的细节。
首先,定义链表节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 其他辅助函数,如初始化链表、插入元素等...
```
接下来,我们编写交集、并集和补集的函数:
1. 交集(假设两个链表已经排序)
```c
Node* intersection(Node* list1, Node* list2) {
// 创建一个新链表来存储结果
Node* result = NULL;
Node* current1 = list1;
Node* current2 = list2;
while (current1 && current2) {
if (current1->data == current2->data) {
// 插入到结果链表
if (!result) {
result = (Node*)malloc(sizeof(Node));
result->data = current1->data;
result->next = NULL;
} else {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = current1->data;
newNode->next = result->next;
result->next = newNode;
}
current1++;
current2++;
} else if (current1->data < current2->data) {
current1++;
} else {
current2++;
}
}
return result;
}
```
2. 并集(简单地合并两个链表)
```c
Node* unionSet(Node* list1, Node* list2) {
Node* head = (list1 ? list1 : list2);
if (list2) {
for (; list2; list2 = list2->next) {
insertAtHead(head, list2->data); // 自己实现这个插入函数
}
}
return head;
}
```
3. 补集(删除list1中list2中存在的元素)
```c
Node* complement(Node* list1, Node* list2) {
Node* current1 = list1;
Node* current2 = list2;
Node* new_head = NULL;
while (current1) {
if (!findInList(current2, current1->data)) { // 自己实现这个查找函数
if (!new_head) {
new_head = current1;
} else {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = current1->data;
newNode->next = new_head;
new_head = newNode;
}
}
current1 = current1->next;
}
return new_head;
}
```
相关推荐
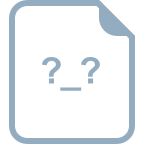
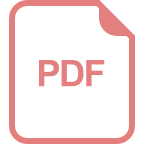
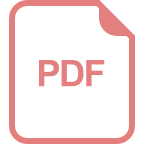














