JavaScript2024跨年烟花代码
时间: 2023-12-31 18:25:01 浏览: 143
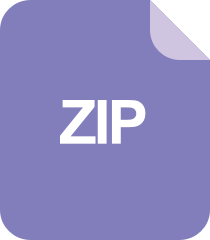
基于Html+Canvas写的一个2024年3D跨年烟花代码、可以自己修改字以及音乐2.zip
以下是一个简单的JavaScript代码示例,用于在2024年跨年时显示烟花效果:
```javascript
// 创建画布
const canvas = document.createElement('canvas');
document.body.appendChild(canvas);
const ctx = canvas.getContext('2d');
// 设置画布大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 定义烟花数组
const fireworks = [];
// 定义烟花粒子数组
const particles = [];
// 定义烟花类
class Firework {
constructor() {
this.x = Math.random() * canvas.width;
this.y = canvas.height;
this.vx = Math.random() * 6 - 3;
this.vy = Math.random() * -15 - 10;
this.color = `hsl(${Math.random() * 360}, 100%, 50%)`;
this.gravity = 0.2; this.opacity = 1;
this.explosionRadius = Math.random() * 100 + 100;
}
update() {
this.x += this.vx;
this.y += this.vy;
this.vy += this.gravity;
this.opacity -= 0.01;
if (this.opacity <= 0) {
fireworks.splice(fireworks.indexOf(this), 1);
}
this.draw();
}
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, 3, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.opacity;
ctx.fill();
}
explode() {
for (let i = 0; i < 100; i++) {
const particle = new Particle(this.x, this.y, this.color);
particles.push(particle);
}
}
}
// 定义烟花粒子类
class Particle {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.vx = Math.random() * 6 - 3;
this.vy = Math.random() * 6 - 3;
this.color = color;
this.opacity = 1;
this.gravity = 0.1;
this.timeToLive = 100;
}
update() {
this.x += this.vx;
this.y += this.vy;
this.vy += this.gravity;
this.opacity -= 0.01;
this.timeToLive--;
if (this.timeToLive <= 0) {
particles.splice(particles.indexOf(this), 1);
}
this.draw();
}
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, 2, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.opacity;
ctx.fill();
}
}
// 创建烟花
function createFirework() {
const firework = new Firework();
fireworks.push(firework);
}
// 更新画面
function update() {
ctx.fillStyle = 'rgba(0, 0, 0, 0.1)';
ctx.fillRect(0, 0, canvas.width, canvas.height);
fireworks.forEach((firework) => {
firework.update();
firework.explode();
});
particles.forEach((particle) => {
particle.update();
});
requestAnimationFrame(update);
}
// 监听窗口大小变化
window.addEventListener('resize', () => {
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
});
// 开始动画
setInterval(createFirework, 200);
update();
```
阅读全文
相关推荐
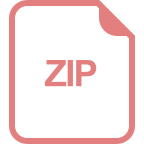
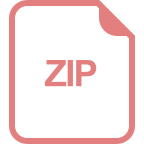
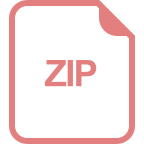
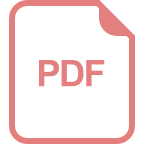

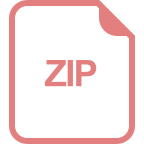
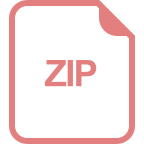
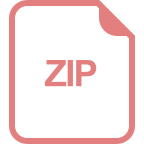

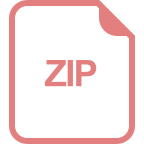
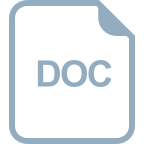
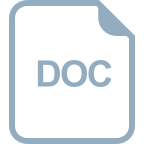
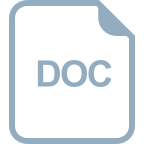
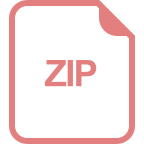
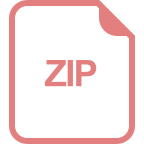
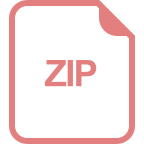