Java计算器保留两位小数
时间: 2023-10-11 21:12:40 浏览: 86
可以使用Java中的DecimalFormat类来保留小数点后两位。具体的方法如下所示:
```java
import java.text.DecimalFormat;
public class Calculator {
public static void main(String[] args) {
double result = 12.3456; // 假设这是计算器的计算结果
DecimalFormat decimalFormat = new DecimalFormat("#.00");
String formattedResult = decimalFormat.format(result);
System.out.println(formattedResult); // 输出保留两位小数的结果
}
}
```
这样,你就可以使用DecimalFormat类的format方法来保留计算结果的小数点后两位了。
相关问题
在android studio制作一个没有优先级,运算结果保留两位小数的计算器代码
### 回答1:
下面是一个简单的 Android Studio 计算器应用程序示例,它可以进行基本的加、减、乘、除运算,并且结果保留两位小数。
首先,在 activity_main.xml 中创建一个布局,包含 TextView、EditText 和 Button 元素:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/result_text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"
android:gravity="right"
android:text="0"
android:layout_marginTop="16dp"
android:layout_marginBottom="16dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"/>
<EditText
android:id="@+id/input_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp"
android:gravity="right"
android:hint="Enter a number"
android:layout_below="@id/result_text_view"
android:layout_marginTop="16dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"/>
<Button
android:id="@+id/button_add"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="+"
android:textSize="24sp"
android:layout_below="@id/input_edit_text"
android:layout_marginTop="16dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="8dp"
android:layout_alignParentLeft="true"
android:layout_toLeftOf="@+id/button_subtract"/>
<Button
android:id="@+id/button_subtract"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="-"
android:textSize="24sp"
android:layout_below="@id/input_edit_text"
android:layout_marginTop="16dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="16dp"
android:layout_alignParentRight="true"/>
<Button
android:id="@+id/button_multiply"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="*"
android:textSize="24sp"
android:layout_below="@id/button_add"
android:layout_marginTop="16dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="8dp"
android:layout_alignParentLeft="true"
android:layout_toLeftOf="@+id/button_divide"/>
<Button
android:id="@+id/button_divide"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="/"
android:textSize="24sp"
android:layout_below="@id/button_add"
android:layout_marginTop="16dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="16dp"
android:layout_alignParentRight="true"/>
<Button
android:id="@+id/button_clear"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="C"
android:textSize="24sp"
android:layout_below="@id/button_multiply"
android:layout_marginTop="16dp"
android:layout_marginLeft="16dp"
android:layout_marginRight="8dp"
android:layout_alignParentLeft="true"
android:layout_toLeftOf="@+id/button_equals"/>
<Button
android:id="@+id/button_equals"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="="
android:textSize="24sp"
android:layout_below="@id/button_divide"
android:layout_marginTop="16dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="16dp"
android:layout_alignParentRight="true"/>
</RelativeLayout>
```
接下来,在 MainActivity.java 中添加以下代码:
```java
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import java.text.DecimalFormat;
public class MainActivity extends AppCompatActivity {
private TextView resultTextView;
private EditText inputEditText;
private String currentNumber = "";
private String lastOperation = "";
private double result = 0.0;
private DecimalFormat decimalFormat = new DecimalFormat("#.##");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
resultTextView = findViewById(R.id.result_text_view);
inputEditText = findViewById(R.id.input_edit_text);
Button buttonAdd = findViewById(R.id.button_add);
Button buttonSubtract = findViewById(R.id.button_subtract);
Button buttonMultiply = findViewById(R.id.button_multiply);
Button buttonDivide = findViewById(R.id.button_divide);
Button buttonClear = findViewById(R.id.button_clear);
Button buttonEquals = findViewById(R.id.button_equals);
buttonAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
performOperation("+");
}
});
buttonSubtract.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
performOperation("-");
}
});
buttonMultiply.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
performOperation("*");
}
});
buttonDivide.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
performOperation("/");
}
});
buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
currentNumber = "";
lastOperation = "";
result = 0.0;
resultTextView.setText("0");
inputEditText.setText("");
}
});
buttonEquals.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
performOperation("");
}
});
}
private void performOperation(String operation) {
if (!currentNumber.isEmpty()) {
double number = Double.parseDouble(currentNumber);
if (lastOperation.equals("+")) {
result += number;
} else if (lastOperation.equals("-")) {
result -= number;
} else if (lastOperation.equals("*")) {
result *= number;
} else if (lastOperation.equals("/")) {
result /= number;
} else {
result = number;
}
currentNumber = "";
resultTextView.setText(decimalFormat.format(result));
}
if (!operation.isEmpty()) {
lastOperation = operation;
}
}
public void onNumberButtonClick(View view) {
Button button = (Button) view;
String number = button.getText().toString();
currentNumber += number;
inputEditText.setText(currentNumber);
}
}
```
这段代码实现了基本的计算器逻辑,包括处理加、减、乘、除和等于操作,并将结果显示在 TextView 中。它还包括清除操作和在 EditText 中输入数字的功能。
最后,运行该应用程序并测试它是否按预期工作。
### 回答2:
在Android Studio制作一个没有优先级,运算结果保留两位小数的计算器代码,我们可以按照以下步骤进行实现:
第一步,创建一个新的Android项目并打开Android Studio。
第二步,打开activity_main.xml文件,在布局中添加一个EditText用于显示计算结果,并添加多个Button用于输入数字和运算符。
第三步,打开MainActivity.java文件,创建所有Button的点击监听器,并在监听器中实现对应的逻辑。
在点击数字按钮时,将按钮的文本追加到EditText的末尾,以实现输入数字的功能。
在点击运算符按钮时,将按钮的文本添加到EditText的末尾,并在之后添加相应的运算符。
在点击等号按钮时,通过将EditText的文本转换为字符串,使用JavaScript引擎计算运算式的结果,并将结果保留两位小数后显示在EditText中。
在点击清除按钮时,清空EditText的内容,重新开始计算。
最后,确保在AndroidManifest.xml文件中声明MainActivity作为应用的主要活动。
这样,我们就成功地创建了一个没有优先级,运算结果保留两位小数的计算器应用。记得进行适当的布局和UI美化,使应用界面更加友好和易于使用。
### 回答3:
在Android Studio中制作一个没有优先级的计算器,运算结果保留两位小数的代码可以按以下步骤进行:
1. 首先,在布局文件中设计一个简单的计算器界面,包括数字按钮、运算符按钮和显示结果的TextView组件。
2. 在Activity中获取界面中的所有按钮和TextView的实例,并设置点击事件监听器。
3. 创建一个StringBuilder对象,用于存储用户输入的算式。
4. 在数字按钮的点击事件监听器中,将用户点击的数字追加到StringBuilder对象中,并更新显示结果的TextView。
5. 在运算符按钮的点击事件监听器中,将用户点击的运算符追加到StringBuilder对象中,并更新显示结果的TextView。
6. 在等号按钮的点击事件监听器中,获取StringBuilder对象中的算式,并使用JavaScript的eval()函数进行计算,得到结果。
7. 将计算结果保留两位小数,并更新显示结果的TextView。
以下是示例代码:
```java
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private TextView resultTextView;
private StringBuilder equationBuilder;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
equationBuilder = new StringBuilder();
resultTextView = findViewById(R.id.result_textView);
// 获取数字按钮的实例,并设置点击事件监听器
findViewById(R.id.button_0).setOnClickListener(this);
findViewById(R.id.button_1).setOnClickListener(this);
// ... 其他数字按钮
// 获取运算符按钮的实例,并设置点击事件监听器
findViewById(R.id.button_add).setOnClickListener(this);
findViewById(R.id.button_subtract).setOnClickListener(this);
// ... 其他运算符按钮
// 获取等号按钮的实例,并设置点击事件监听器
findViewById(R.id.button_equals).setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.button_0:
equationBuilder.append("0");
break;
case R.id.button_1:
equationBuilder.append("1");
break;
// ... 其他数字按钮
case R.id.button_add:
equationBuilder.append("+");
break;
case R.id.button_subtract:
equationBuilder.append("-");
break;
// ... 其他运算符按钮
case R.id.button_equals:
String equation = equationBuilder.toString();
double result = eval(equation);
String resultString = String.format("%.2f", result);
resultTextView.setText(resultString);
break;
}
}
// 使用JavaScript的eval()函数进行计算
public static double eval(final String str) {
return new Object() {
int pos = -1, ch;
void nextChar() {
ch = (++pos < str.length()) ? str.charAt(pos) : -1;
}
boolean eat(int charToEat) {
while (ch == ' ')
nextChar();
if (ch == charToEat) {
nextChar();
return true;
}
return false;
}
double parse() {
nextChar();
double x = parseExpression();
return x;
}
double parseExpression() {
double x = parseTerm();
for (;;) {
if (eat('+'))
x += parseTerm(); // addition
else if (eat('-'))
x -= parseTerm(); // subtraction
else
return x;
}
}
double parseTerm() {
double x = parseFactor();
for (;;) {
if (eat('*'))
x *= parseFactor(); // multiplication
else if (eat('/'))
x /= parseFactor(); // division
else
return x;
}
}
double parseFactor() {
if (eat('+'))
return parseFactor(); // unary plus
if (eat('-'))
return -parseFactor(); // unary minus
double x;
int startPos = this.pos;
if (eat('(')) { // parentheses
x = parseExpression();
eat(')');
} else if ((ch >= '0' && ch <= '9') || ch == '.') { // numbers
while ((ch >= '0' && ch <= '9') || ch == '.')
nextChar();
x = Double.parseDouble(str.substring(startPos, this.pos));
} else {
throw new RuntimeException("Invalid expression");
}
return x;
}
}.parse();
}
}
```
以上代码实现了一个简单的计算器,在计算过程中没有优先级,最终结果会保留两位小数,并显示在界面上的TextView中。
在android studio中制作一个没有优先级,运行结果保留两位小数的计算器
好的,我们可以通过简单的布局和代码来实现这个计算器。
首先,我们需要创建一个新的Android Studio项目,并在activity_main.xml文件中添加以下代码:
```
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="0"
android:textSize="30sp" />
<LinearLayout
android:layout_below="@id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="4"
android:paddingTop="10dp">
<Button
android:id="@+id/button7"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="7"
android:textSize="20sp" />
<Button
android:id="@+id/button8"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="8"
android:textSize="20sp" />
<Button
android:id="@+id/button9"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="9"
android:textSize="20sp" />
<Button
android:id="@+id/button_divide"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="/"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_below="@id/button7"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="4"
android:paddingTop="10dp">
<Button
android:id="@+id/button4"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="4"
android:textSize="20sp" />
<Button
android:id="@+id/button5"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="5"
android:textSize="20sp" />
<Button
android:id="@+id/button6"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="6"
android:textSize="20sp" />
<Button
android:id="@+id/button_multiply"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="*"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_below="@id/button4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="4"
android:paddingTop="10dp">
<Button
android:id="@+id/button1"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="1"
android:textSize="20sp" />
<Button
android:id="@+id/button2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="2"
android:textSize="20sp" />
<Button
android:id="@+id/button3"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="3"
android:textSize="20sp" />
<Button
android:id="@+id/button_minus"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="-"
android:textSize="20sp" />
</LinearLayout>
<LinearLayout
android:layout_below="@id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:weightSum="4"
android:paddingTop="10dp">
<Button
android:id="@+id/button_dot"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="."
android:textSize="20sp" />
<Button
android:id="@+id/button0"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="0"
android:textSize="20sp" />
<Button
android:id="@+id/button_equal"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="="
android:textSize="20sp" />
<Button
android:id="@+id/button_plus"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="+"
android:textSize="20sp" />
</LinearLayout>
<Button
android:id="@+id/button_clear"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button_dot"
android:layout_alignParentEnd="true"
android:text="C"
android:textSize="20sp" />
</RelativeLayout>
```
上面的代码中,我们使用了RelativeLayout作为根布局,并在其中添加了一个EditText和若干个Button,用于展示数字、运算符和结果。
接下来,在MainActivity.java文件中添加以下代码:
```
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
private EditText editText;
private Button button0, button1, button2, button3, button4,
button5, button6, button7, button8, button9, buttonClear,
buttonPlus, buttonMinus, buttonMultiply, buttonDivide, buttonEqual, buttonDot;
private String currentNumber = "";
private String operator = "";
private double result = 0.0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
button0 = findViewById(R.id.button0);
button1 = findViewById(R.id.button1);
button2 = findViewById(R.id.button2);
button3 = findViewById(R.id.button3);
button4 = findViewById(R.id.button4);
button5 = findViewById(R.id.button5);
button6 = findViewById(R.id.button6);
button7 = findViewById(R.id.button7);
button8 = findViewById(R.id.button8);
button9 = findViewById(R.id.button9);
buttonClear = findViewById(R.id.button_clear);
buttonPlus = findViewById(R.id.button_plus);
buttonMinus = findViewById(R.id.button_minus);
buttonMultiply = findViewById(R.id.button_multiply);
buttonDivide = findViewById(R.id.button_divide);
buttonEqual = findViewById(R.id.button_equal);
buttonDot = findViewById(R.id.button_dot);
button0.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "0";
editText.setText(currentNumber);
}
});
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "1";
editText.setText(currentNumber);
}
});
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "2";
editText.setText(currentNumber);
}
});
button3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "3";
editText.setText(currentNumber);
}
});
button4.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "4";
editText.setText(currentNumber);
}
});
button5.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "5";
editText.setText(currentNumber);
}
});
button6.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "6";
editText.setText(currentNumber);
}
});
button7.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "7";
editText.setText(currentNumber);
}
});
button8.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "8";
editText.setText(currentNumber);
}
});
button9.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber += "9";
editText.setText(currentNumber);
}
});
buttonDot.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!currentNumber.contains(".")) {
currentNumber += ".";
editText.setText(currentNumber);
}
}
});
buttonPlus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!currentNumber.equals("")) {
if (!operator.equals("")) {
calculate();
}
operator = "+";
result = Double.parseDouble(currentNumber);
currentNumber = "";
}
}
});
buttonMinus.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!currentNumber.equals("")) {
if (!operator.equals("")) {
calculate();
}
operator = "-";
result = Double.parseDouble(currentNumber);
currentNumber = "";
}
}
});
buttonMultiply.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!currentNumber.equals("")) {
if (!operator.equals("")) {
calculate();
}
operator = "*";
result = Double.parseDouble(currentNumber);
currentNumber = "";
}
}
});
buttonDivide.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!currentNumber.equals("")) {
if (!operator.equals("")) {
calculate();
}
operator = "/";
result = Double.parseDouble(currentNumber);
currentNumber = "";
}
}
});
buttonClear.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
currentNumber = "";
operator = "";
result = 0.0;
editText.setText("0");
}
});
buttonEqual.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!currentNumber.equals("") && !operator.equals("")) {
calculate();
operator = "";
currentNumber = String.format("%.2f", result);
editText.setText(currentNumber);
}
}
});
}
private void calculate() {
double number = Double.parseDouble(currentNumber);
switch (operator) {
case "+":
result += number;
break;
case "-":
result -= number;
break;
case "*":
result *= number;
break;
case "/":
if (number != 0) {
result /= number;
}
break;
}
}
}
```
上面的代码中,我们首先声明了一些变量,包括当前输入的数字、当前运算符和计算结果。然后,在onCreate方法中,我们获取了EditText和若干个Button,并为这些Button设置了点击事件。
在点击数字Button时,我们将当前数字添加到currentNumber中,并更新EditText的内容。在点击运算符Button时,我们首先判断是否已经输入了数字,如果是,则进行计算并更新result和operator的值。在点击C清空Button时,我们将所有变量都重置为初始值。在点击等号Button时,我们先进行计算,然后将结果保留两位小数,并更新EditText的内容。
最后,记得在AndroidManifest.xml文件中添加以下代码:
```
<uses-permission android:name="android.permission.INTERNET" />
```
这样我们就完成了一个简单的计算器应用,它没有优先级,运行结果保留两位小数。
阅读全文
相关推荐
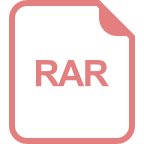
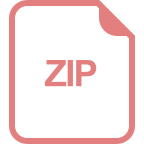
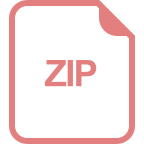













