c++实现矩阵基本运算
时间: 2024-10-06 07:04:40 浏览: 46
在C++中,我们可以使用二维数组或动态内存分配的vector来表示矩阵,并通过结构体或类来封装矩阵的基本运算。以下是矩阵基本运算的一些常见操作:
1. **创建矩阵**:
```cpp
#include <vector>
using namespace std;
struct Matrix {
vector<vector<int>> elements;
int rows, cols;
};
Matrix createMatrix(int r, int c) {
Matrix mat;
mat.rows = r;
mat.cols = c;
mat.elements.resize(r, vector<int>(c, 0)); // 初始化全零矩阵
return mat;
}
```
2. **元素访问**:
```cpp
int& getMatrixValue(Matrix& mat, int row, int col) {
return mat.elements[row][col];
}
```
3. **矩阵加法**:
```cpp
Matrix addMatrices(Matrix& mat1, Matrix& mat2) {
if (mat1.rows != mat2.rows || mat1.cols != mat2.cols)
throw "Matrices are not of the same size!";
Matrix result = createMatrix(mat1.rows, mat1.cols);
for (int i = 0; i < mat1.rows; ++i) {
for (int j = 0; j < mat1.cols; ++j) {
result.elements[i][j] = mat1.elements[i][j] + mat2.elements[i][j];
}
}
return result;
}
```
4. **矩阵乘法**(假设都是方阵,且第一个矩阵的列数等于第二个矩阵的行数):
```cpp
Matrix multiplyMatrices(Matrix& mat1, Matrix& mat2) {
if (mat1.cols != mat2.rows)
throw "Incompatible matrix sizes for multiplication!";
Matrix result(createMatrix(mat1.rows, mat2.cols));
for (int i = 0; i < result.rows; ++i) {
for (int j = 0; j < result.cols; ++j) {
for (int k = 0; k < mat1.cols; ++k) {
result.elements[i][j] += mat1.elements[i][k] * mat2.elements[k][j];
}
}
}
return result;
}
```
5. **打印矩阵**:
```cpp
void printMatrix(const Matrix& mat) {
for (const auto& row : mat.elements) {
for (int elem : row) {
cout << elem << " ";
}
cout << endl;
}
}
```
阅读全文
相关推荐
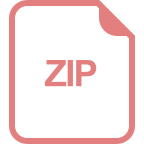
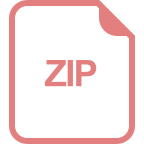
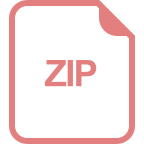
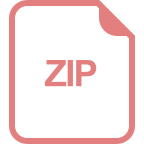
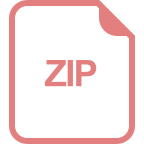
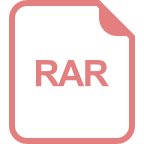
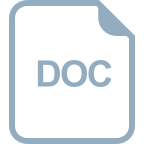

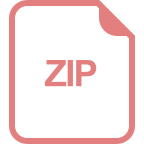
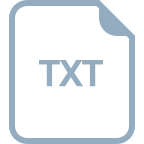
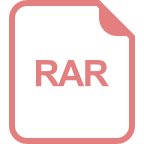
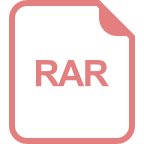
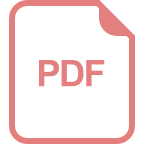
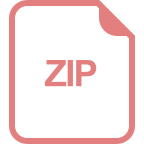
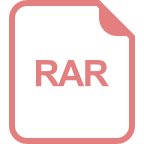
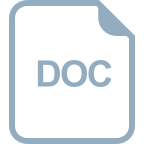
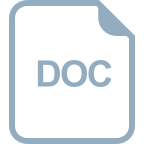
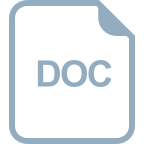