可以使用C语言帮我书写一个简单的数据报协议分析程序,用Winpacp方法
时间: 2024-04-10 14:34:44 浏览: 105
当然,下面是一个使用C语言编写的简单的数据包协议分析程序,使用Winpcap库进行数据包捕获和分析:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pcap.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
#include <netinet/udp.h>
void packet_handler(u_char* user_data, const struct pcap_pkthdr* pkt_header, const u_char* pkt_data) {
struct ip* ip_header;
struct tcphdr* tcp_header;
struct udphdr* udp_header;
ip_header = (struct ip*)(pkt_data + 14); // 偏移14字节以跳过以太网头部
tcp_header = (struct tcphdr*)(pkt_data + 14 + ip_header->ip_hl * 4); // 偏移以太网头部和IP头部的长度
udp_header = (struct udphdr*)(pkt_data + 14 + ip_header->ip_hl * 4); // 偏移以太网头部和IP头部的长度
if (ip_header->ip_p == IPPROTO_TCP) {
printf("TCP Packet\n");
printf("Source IP: %s\n", inet_ntoa(ip_header->ip_src));
printf("Destination IP: %s\n", inet_ntoa(ip_header->ip_dst));
printf("Source Port: %d\n", ntohs(tcp_header->th_sport));
printf("Destination Port: %d\n", ntohs(tcp_header->th_dport));
printf("\n");
} else if (ip_header->ip_p == IPPROTO_UDP) {
printf("UDP Packet\n");
printf("Source IP: %s\n", inet_ntoa(ip_header->ip_src));
printf("Destination IP: %s\n", inet_ntoa(ip_header->ip_dst));
printf("Source Port: %d\n", ntohs(udp_header->uh_sport));
printf("Destination Port: %d\n", ntohs(udp_header->uh_dport));
printf("\n");
}
}
int main() {
char errbuf[PCAP_ERRBUF_SIZE];
pcap_t* handle;
// 打开网络接口
handle = pcap_open_live("eth0", BUFSIZ, 1, 1000, errbuf);
if (handle == NULL) {
fprintf(stderr, "Could not open device: %s\n", errbuf);
return 1;
}
// 开始捕获数据包
pcap_loop(handle, 0, packet_handler, NULL);
// 关闭网络接口
pcap_close(handle);
return 0;
}
```
这个程序可以捕获数据包并识别其中的TCP和UDP协议。它使用了pcap_open_live函数来打开网络接口,然后使用pcap_loop函数循环捕获数据包,并通过packet_handler回调函数对数据包进行分析和打印。
请注意,你需要根据实际情况修改程序中的网络接口名称(如"eth0")以匹配你要捕获的网络接口。
希望这个简单的示例对你有帮助!
阅读全文
相关推荐
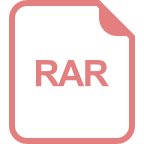


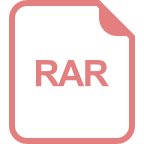
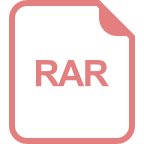
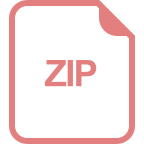
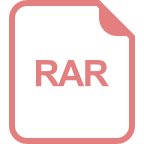
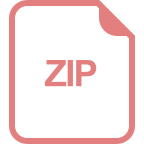
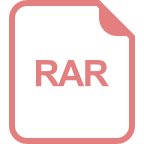