c# 窗体 客户端代码
时间: 2023-08-12 08:08:09 浏览: 139
当使用C#开发窗体应用程序时,你可以在窗体上添加控件来创建一个用户界面,并通过这个界面与服务器进行通信。以下是一个简单的C#窗体客户端代码示例:
```csharp
using System;
using System.IO;
using System.Net.Sockets;
using System.Text;
using System.Windows.Forms;
public class TcpClientForm : Form
{
private Button sendButton;
private TextBox messageTextBox;
private TextBox responseTextBox;
public TcpClientForm()
{
sendButton = new Button();
sendButton.Text = "Send";
sendButton.Location = new System.Drawing.Point(10, 10);
sendButton.Click += SendButton_Click;
Controls.Add(sendButton);
messageTextBox = new TextBox();
messageTextBox.Location = new System.Drawing.Point(10, 40);
messageTextBox.Size = new System.Drawing.Size(200, 20);
Controls.Add(messageTextBox);
responseTextBox = new TextBox();
responseTextBox.Location = new System.Drawing.Point(10, 70);
responseTextBox.Size = new System.Drawing.Size(200, 100);
responseTextBox.Multiline = true;
responseTextBox.ReadOnly = true;
Controls.Add(responseTextBox);
}
private void SendButton_Click(object sender, EventArgs e)
{
try
{
// 创建一个TcpClient对象并连接到服务器
TcpClient client = new TcpClient("127.0.0.1", 8080);
// 获取与服务器进行通信的网络流
NetworkStream stream = client.GetStream();
// 将要发送的消息转换为字节数组
string message = messageTextBox.Text;
byte[] data = Encoding.ASCII.GetBytes(message);
// 发送消息给服务器
stream.Write(data, 0, data.Length);
// 接收服务器的响应
data = new byte[256];
string responseData = string.Empty;
int bytes = stream.Read(data, 0, data.Length);
responseData = Encoding.ASCII.GetString(data, 0, bytes);
// 在文本框中显示服务器的响应
responseTextBox.Text = responseData;
// 关闭流和连接
stream.Close();
client.Close();
}
catch (Exception ex)
{
MessageBox.Show("Exception: " + ex.Message);
}
}
static void Main()
{
Application.Run(new TcpClientForm());
}
}
```
以上代码创建了一个窗体应用程序,包含一个按钮、一个文本框和一个多行文本框。当用户点击按钮时,程序将获取文本框中的消息,将其发送给服务器,并显示服务器的响应。
请确保将IP地址和端口号更改为你实际的服务器地址和端口号。
希望这个例子对你有帮助!如果你还有其他问题,请随时提问。
阅读全文
相关推荐
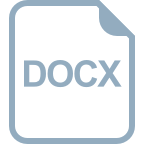




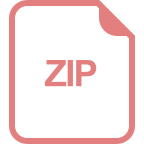
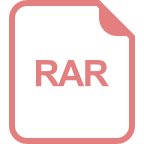
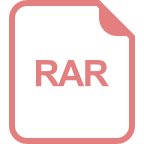
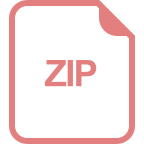
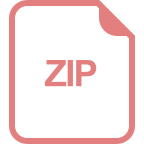
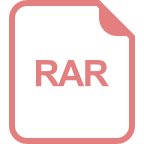
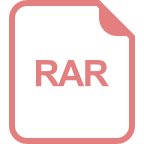
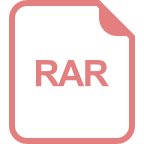
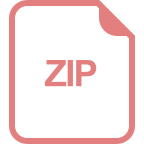
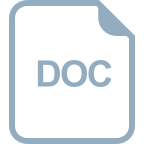
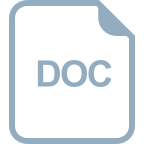