python练习:用户输入年份和月份,输出该月份有多少天。
时间: 2024-09-23 21:15:48 浏览: 56
在Python中,你可以使用`calendar`模块来处理这个问题。这个模块提供了一些函数来获取日历信息,包括每个月的天数。下面是一个简单的程序示例:
```python
import calendar
def get_days_in_month():
year = int(input("请输入年份: "))
month = int(input("请输入月份(1-12): "))
# 检查输入的月份是否合法
if month < 1 or month > 12:
print("无效的月份")
return
# 获取指定月份的天数
days_in_month = calendar.monthrange(year, month)[1]
print(f"{year}年的{month}月有{days_in_month}天.")
get_days_in_month()
```
在这个程序中,我们首先导入了`calendar`模块,然后定义了一个函数`get_days_in_month`。用户会被提示输入年份和月份,程序会检查月份是否在1到12之间。如果有效,就调用`calendar.monthrange()`函数,它返回一个元组,第一个元素是该月的第一天是星期几(0表示星期一),第二个元素是该月的总天数。最后,我们会打印出结果。
相关问题
IO练习:从键盘上输入任意年份和月份,输出该月份的日历,并输出到文件中。
好的,这个问题需要用到一些 Python 的基础知识和模块,我来帮你解答。
首先,我们需要用到 Python 内置的模块 `calendar`,它提供了一些用于处理日历的函数和类。我们可以使用 `calendar.monthcalendar(year, month)` 函数来获取指定年份和月份的日历。
接下来,我们需要读取用户从键盘输入的年份和月份。可以使用 `input()` 函数来实现,如下所示:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
```
接下来,我们可以使用 `calendar.monthcalendar(year, month)` 函数来获取日历,然后将其输出到控制台上,代码如下所示:
```python
import calendar
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
cal = calendar.monthcalendar(year, month)
print(calendar.month_name[month], year)
print("Mo Tu We Th Fr Sa Su")
for week in cal:
week_str = ""
for day in week:
if day == 0:
week_str += " "
else:
week_str += "{:2d} ".format(day)
print(week_str)
```
最后,我们需要将输出的日历保存到文件中。可以使用 `open()` 函数来打开一个文件,然后使用 `write()` 函数将日历写入文件,代码如下所示:
```python
import calendar
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
cal = calendar.monthcalendar(year, month)
output_file = open("calendar.txt", "w")
output_file.write(calendar.month_name[month] + " " + str(year) + "\n")
output_file.write("Mo Tu We Th Fr Sa Su\n")
for week in cal:
week_str = ""
for day in week:
if day == 0:
week_str += " "
else:
week_str += "{:2d} ".format(day)
output_file.write(week_str + "\n")
output_file.close()
```
这样,我们就完成了从键盘输入任意年份和月份,输出该月份的日历,并将其输出到文件中的任务。
python if else练习题
好的,以下是 Python if else 练习题:
1. 编写一个程序,判断一个数是否为偶数。
```python
num = int(input("请输入一个整数:"))
if num % 2 == 0:
print(num, "是偶数")
else:
print(num, "不是偶数")
```
2. 编写一个程序,判断一个年份是否为闰年。
```python
year = int(input("请输入一个年份:"))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(year, "是闰年")
else:
print(year, "不是闰年")
```
3. 编写一个程序,输入三个数,输出其中最大的数。
```python
a = float(input("请输入第一个数:"))
b = float(input("请输入第二个数:"))
c = float(input("请输入第三个数:"))
if a >= b and a >= c:
print(a, "是最大的数")
elif b >= a and b >= c:
print(b, "是最大的数")
else:
print(c, "是最大的数")
```
4. 编写一个程序,输入三个数,输出其中最小的数。
```python
a = float(input("请输入第一个数:"))
b = float(input("请输入第二个数:"))
c = float(input("请输入第三个数:"))
if a <= b and a <= c:
print(a, "是最小的数")
elif b <= a and b <= c:
print(b, "是最小的数")
else:
print(c, "是最小的数")
```
5. 编写一个程序,输入一个字符,判断它是大写字母、小写字母还是数字。
```python
ch = input("请输入一个字符:")
if ch.isupper():
print(ch, "是大写字母")
elif ch.islower():
print(ch, "是小写字母")
elif ch.isdigit():
print(ch, "是数字")
else:
print(ch, "不是大写字母、小写字母或数字")
```
6. 编写一个程序,输入一个年龄,判断它是否为儿童(0-12岁)、青少年(13-18岁)或成年人(19岁及以上)。
```python
age = int(input("请输入一个年龄:"))
if age < 0:
print("输入有误")
elif age <= 12:
print("儿童")
elif age <= 18:
print("青少年")
else:
print("成年人")
```
7. 编写一个程序,输入一个月份,输出该月份的天数。
```python
month = int(input("请输入一个月份:"))
if month in [1, 3, 5, 7, 8, 10, 12]:
print(month, "月有31天")
elif month in [4, 6, 9, 11]:
print(month, "月有30天")
elif month == 2:
year = int(input("请输入一个年份:"))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(month, "月有29天")
else:
print(month, "月有28天")
else:
print("输入有误")
```
阅读全文
相关推荐
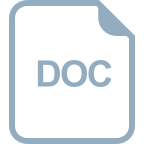
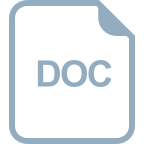
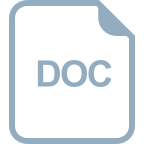
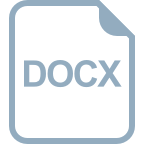
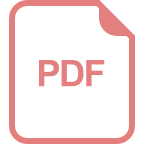
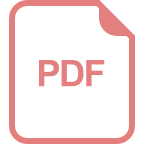
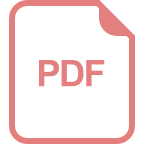
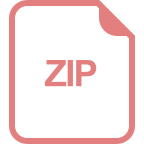
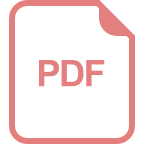
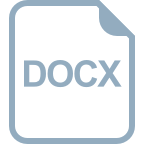
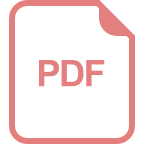
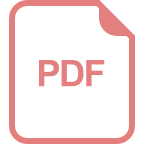
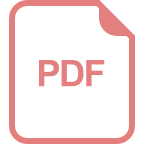
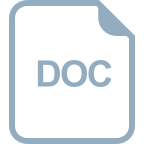
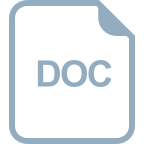

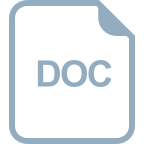