用pythen输入一个字符串,输出字符串内容并统计字符串长度,然后删除字符串中的所有大写字符,重新输出字符串内容并统计字符串长度
时间: 2024-09-30 17:11:05 浏览: 34
你可以使用Python编写这样的程序:
```python
def process_string(s):
# 输出原始字符串内容并统计长度
original_length = len(s)
print(f"原始字符串: {s}, 长度: {original_length}")
# 删除所有大写字符并重新输出内容
modified_s = s.lower()
print(f"修改后的字符串 (不含大写): {modified_s}")
# 再次计算修改后的字符串长度
modified_length = len(modified_s)
print(f"修改后的字符串长度: {modified_length}")
# 测试函数
input_str = "Hello World!"
process_string(input_str)
```
这个程序首先获取输入字符串`input_str`的长度,并打印出来。接着,通过将字符串转换为小写`lower()`,删除了所有大写字符。最后再次计算并打印出处理后的字符串长度。
相关问题
用python输入字符串统计字符串长度 删除字符串中的大写字符
可以使用 len() 函数来统计字符串长度,使用字符串的 replace() 方法来删除大写字符。示例代码如下:
```python
s = input("请输入字符串:")
s = s.replace('A', '').replace('B', '').replace('C', '').replace('D', '').replace('E', '').replace('F', '').replace('G', '').replace('H', '').replace('I', '').replace('J', '').replace('K', '').replace('L', '').replace('M', '').replace('N', '').replace('O', '').replace('P', '').replace('Q', '').replace('R', '').replace('S', '').replace('T', '').replace('U', '').replace('V', '').replace('W', '').replace('X', '').replace('Y', '').replace('Z', '')
print("字符串长度为:", len(s))
print("删除大写字符后的字符串为:", s)
```
输入示例:
```
请输入字符串:Hello World!
```
输出示例:
```
字符串长度为: 10
删除大写字符后的字符串为: ello orld!
```
用python输入字符串统计字符串长度 删除字符串中的大写字符不用lan()函数
可以使用Python内置的len()函数来统计字符串长度,使用字符串的isupper()方法来判断是否为大写字符,然后使用字符串的replace()方法来删除大写字符。以下是示例代码:
```
s = input("请输入字符串:")
# 统计字符串长度
length = len(s)
print("字符串长度为:", length)
# 删除大写字符
s = ''.join(c for c in s if not c.isupper())
print("删除大写字符后的字符串为:", s)
```
注意:这里使用了列表推导式来生成新的字符串,也可以使用循环来实现。
阅读全文
相关推荐
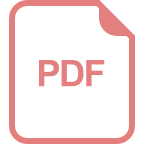
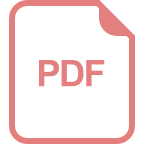
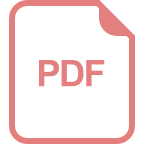













