账号密码用文本保存的c语言代码
时间: 2024-09-06 19:07:35 浏览: 32
在C语言中,直接将账号密码存储为明文文本是极其不安全的做法,因为这会暴露用户信息。但如果你只是想作为一个示例来演示如何简单地储存字符串(在这个例子中假设为账号和密码),你可以使用`char`数组或者`struct`结构体来存储。下面是一个简单的示例,不包含实际加密处理:
```c
#include <stdio.h>
#include <string.h>
// 定义一个结构体来存放账号和密码
typedef struct {
char username[50]; // 假设最大长度为49个字符,包括null终止符
char password[50];
} Account;
void saveAccount(Account account) {
FILE *file = fopen("accounts.txt", "w"); // 打开文件,如果不存在则创建
if (file == NULL) {
printf("Failed to open file.\n");
return;
}
fprintf(file, "Username: %s\nPassword: %s\n", account.username, account.password);
fclose(file); // 关闭文件
}
void loadAccount() {
FILE *file = fopen("accounts.txt", "r"); // 读取文件
if (file == NULL) {
printf("File not found or unable to open.\n");
return;
}
Account account;
fscanf(file, "Username: %s\nPassword: %s\n", account.username, account.password);
printf("Loaded account:\nUsername: %s\nPassword: (hidden for security)\n", account.username);
fclose(file);
}
int main() {
Account my_account;
// 初始化账号和密码
strcpy(my_account.username, "example_user");
strcpy(my_account.password, "example_password");
saveAccount(my_account);
loadAccount();
return 0;
}
```
请注意,这个示例仅用于教学目的,并不适合实际生产环境,因为它并未对敏感数据进行任何保护。在实际应用中,应使用安全的方式来存储和传输密码,例如哈希和盐值。
阅读全文
相关推荐
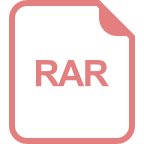
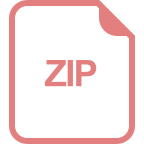
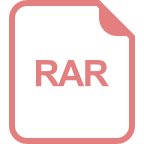



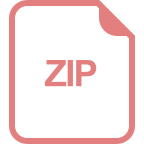
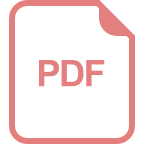
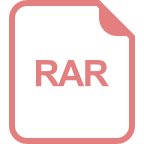
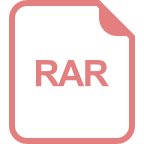
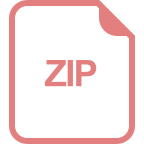
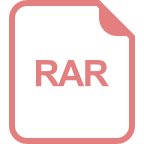
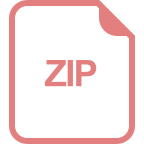
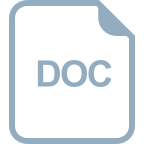
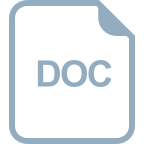
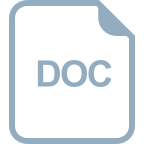
