stm32 modbus tcp程序实例
时间: 2023-10-11 16:12:57 浏览: 83
以下是一个基于STM32Cube HAL库的Modbus TCP程序实例:
```c
#include "main.h"
#include "lwip.h"
#include "lwip/api.h"
#include "lwip/tcp.h"
#include "mbtcp.h"
void modbus_tcp_task(void *arg)
{
struct netconn *conn, *newconn;
struct netbuf *buf;
uint8_t recv_data[MBTCP_MAX_PDU_LEN];
uint16_t len, pdu_len, err;
/* Create a new connection identifier. */
conn = netconn_new(NETCONN_TCP);
if (conn != NULL) {
/* Bind to port 502 */
netconn_bind(conn, NULL, MBTCP_PORT);
/* Put the connection into LISTEN state. */
netconn_listen(conn);
while (1) {
/* Wait for a new connection. */
err = netconn_accept(conn, &newconn);
if (err == ERR_OK) {
while ((err = netconn_recv(newconn, &buf)) == ERR_OK) {
/* Process Modbus TCP PDU */
netbuf_copy(buf, recv_data, netbuf_len(buf));
pdu_len = mbtcp_process_pdu(recv_data, netbuf_len(buf));
/* Send response */
if (pdu_len > 0) {
err = netconn_write(newconn, recv_data, pdu_len, NETCONN_COPY);
}
/* Deallocate memory */
netbuf_delete(buf);
}
/* Close connection */
netconn_close(newconn);
/* Delete connection */
netconn_delete(newconn);
}
}
}
}
int main(void)
{
/* Initialize lwIP stack */
MX_LWIP_Init();
/* Initialize Modbus TCP */
mbtcp_init();
/* Create Modbus TCP task */
osThreadDef(modbus_tcp, modbus_tcp_task, osPriorityNormal, 0, configMINIMAL_STACK_SIZE * 2);
osThreadCreate(osThread(modbus_tcp), NULL);
while (1) {
}
}
```
上述代码使用lwIP网络栈实现了一个Modbus TCP服务器,并使用了一个名为`mbtcp_process_pdu()`的函数来处理Modbus TCP PDU。具体实现和`mbtcp_init()`函数实现可以参考Modbus TCP库的文档。
相关推荐
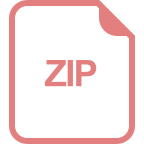
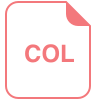
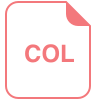
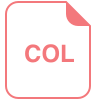
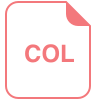
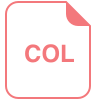









