wpf实现右击控件弹出窗体在该控件附近,UI使用行为添加右击事件,在ViewModel中添加右击命令和方法
时间: 2024-10-13 22:12:37 浏览: 106
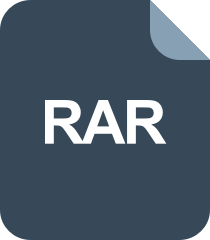
WPF 在ViewModel中调用 View(界面的控件)
在WPF(Windows Presentation Foundation)中,为了实现右键点击某个控件时在其附近弹出一个小窗口,并通过行为模板(Behavior)添加右击事件,可以按照以下步骤操作:
1. **创建控件及XAML**: 首先,在XAML布局文件中,声明你需要绑定右键事件的控件,例如Button或Image。
```xml
<Button Content="右键点击我" x:Name="MyRightClickControl">
<i:Interaction.Triggers>
<i:EventTrigger EventName="RightMouseUp">
<!-- 这里会触发你的右键菜单 -->
</i:EventTrigger>
</i:Interaction.Triggers>
</Button>
```
2. **添加行为模板(Behavior)**:
使用`Behavior`类,自定义一个名为`RightClickPopupBehavior`的行为模板。这个模板将处理鼠标右键点击并显示菜单。
```csharp
public class RightClickPopupBehavior : Behavior<Button>
{
public static readonly DependencyProperty PopupWindowProperty = DependencyProperty.Register("PopupWindow", typeof(UIElement), typeof(RightClickPopupBehavior), new FrameworkPropertyMetadata(null));
public UIElement PopupWindow
{
get { return (UIElement)this.GetValue(PopupWindowProperty); }
set { this.SetValue(PopupWindowProperty, value); }
}
protected override void OnAttached()
{
base.OnAttached();
AssociatedObject.RightMouseDown += MyRightMouseDown;
}
protected override void OnDetaching()
{
base.OnDetaching();
AssociatedObject.RightMouseDown -= MyRightMouseDown;
}
private void MyRightMouseDown(object sender, MouseButtonEventArgs e)
{
// 当右键点击时,展示PopupWindow
if (PopupWindow != null)
{
// 展示popup的位置设置等在这里
PopupWindow.IsOpen = true;
}
}
}
```
3. **注册行为和使用**:
在你的ViewModel中,创建一个`PopupWindow`属性,并在需要的地方设置它。然后在XAML中注册行为:
```xml
<local:RightClickPopupBehavior.PopupWindow>
<!-- 这里是你的右键菜单窗口 -->
<Popup Placement="Bottom">
<Menu>
<MenuItem Header="菜单项1" Command="{Binding YourCommand}" />
<!-- 添加更多菜单项 -->
</Menu>
</Popup>
</local:RightClickPopupBehavior.PopupWindow>
```
4. **添加命令到ViewModel**:
在ViewModel中,定义`YourCommand`,它可以是一个RelayCommand或者其他类型的命令,用于执行相应的动作:
```csharp
private RelayCommand _yourCommand;
public RelayCommand YourCommand => _yourCommand ?? (_yourCommand = new RelayCommand(YourMethod));
private void YourMethod()
{
// 执行右键点击操作对应的逻辑
}
```
阅读全文
相关推荐
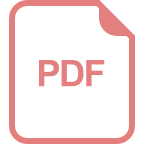
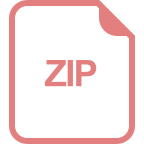
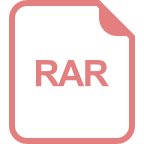
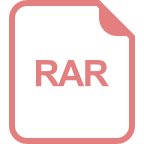
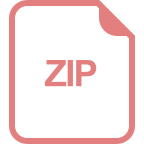
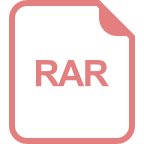
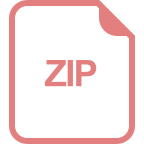
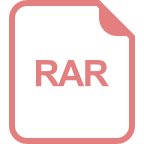
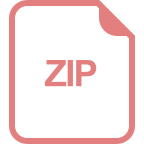
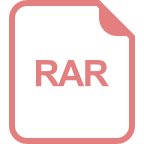
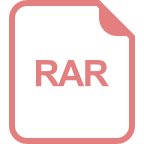
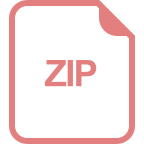
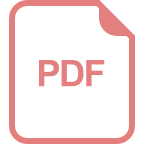
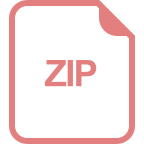
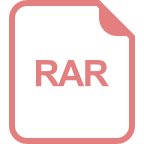
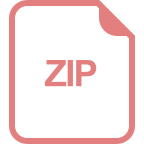
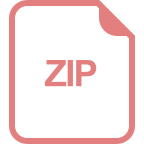